java实现五子棋游戏可开始,认输,悔棋
时间: 2023-12-09 19:06:27 浏览: 44
好的,以下是Java实现五子棋游戏的示例代码,包括可开始、认输、悔棋等功能:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Gobang extends JFrame implements ActionListener, MouseListener {
private static final long serialVersionUID = 1L;
private JPanel mainPanel;
private int[][] chessboard;
private int currentPlayer;
private JLabel statusLabel;
private JButton restartButton;
private JButton backButton;
private int currentX;
private int currentY;
private int winner;
public Gobang() {
super("五子棋游戏");
mainPanel = new JPanel(new GridLayout(15, 15));
chessboard = new int[15][15];
currentPlayer = 1;
winner = 0;
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
JPanel panel = new JPanel();
panel.setBorder(BorderFactory.createLineBorder(Color.BLACK));
mainPanel.add(panel);
panel.addMouseListener(this);
}
}
statusLabel = new JLabel("当前玩家: " + currentPlayer);
restartButton = new JButton("重新开始");
backButton = new JButton("悔棋");
JPanel bottomPanel = new JPanel(new GridLayout(1, 3));
bottomPanel.add(statusLabel);
bottomPanel.add(restartButton);
bottomPanel.add(backButton);
restartButton.addActionListener(this);
backButton.addActionListener(this);
add(mainPanel, BorderLayout.CENTER);
add(bottomPanel, BorderLayout.SOUTH);
pack();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == restartButton) {
restart();
} else if (e.getSource() == backButton) {
if (currentPlayer != winner) {
undo();
}
}
}
public void mouseClicked(MouseEvent e) {
if (winner != 0) {
return;
}
JPanel panel = (JPanel) e.getSource();
int index = mainPanel.getComponentZOrder(panel);
int x = index % 15;
int y = index / 15;
if (chessboard[x][y] != 0) {
return;
}
currentX = x;
currentY = y;
chessboard[x][y] = currentPlayer;
panel.setBackground(currentPlayer == 1 ? Color.BLACK : Color.WHITE);
if (checkWin(x, y)) {
winner = currentPlayer;
statusLabel.setText("玩家 " + winner + " 获胜");
} else {
currentPlayer = currentPlayer == 1 ? 2 : 1;
statusLabel.setText("当前玩家: " + currentPlayer);
}
}
public void mousePressed(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
private boolean checkWin(int x, int y) {
int count = 1;
// 横向
for (int i = x - 1; i >= 0; i--) {
if (chessboard[i][y] == currentPlayer) {
count++;
} else {
break;
}
}
for (int i = x + 1; i < 15; i++) {
if (chessboard[i][y] == currentPlayer) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
count = 1;
// 纵向
for (int i = y - 1; i >= 0; i--) {
if (chessboard[x][i] == currentPlayer) {
count++;
} else {
break;
}
}
for (int i = y + 1; i < 15; i++) {
if (chessboard[x][i] == currentPlayer) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
count = 1;
// 斜向
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0; i--, j--) {
if (chessboard[i][j] == currentPlayer) {
count++;
} else {
break;
}
}
for (int i = x + 1, j = y + 1; i < 15 && j < 15; i++, j++) {
if (chessboard[i][j] == currentPlayer) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
count = 1;
// 反斜向
for (int i = x - 1, j = y + 1; i >= 0 && j < 15; i--, j++) {
if (chessboard[i][j] == currentPlayer) {
count++;
} else {
break;
}
}
for (int i = x + 1, j = y - 1; i < 15 && j >= 0; i++, j--) {
if (chessboard[i][j] == currentPlayer) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
return false;
}
private void undo() {
chessboard[currentX][currentY] = 0;
Component component = mainPanel.getComponent(currentY * 15 + currentX);
component.setBackground(null);
currentPlayer = currentPlayer == 1 ? 2 : 1;
statusLabel.setText("当前玩家: " + currentPlayer);
}
private void restart() {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
chessboard[i][j] = 0;
Component component = mainPanel.getComponent(j * 15 + i);
component.setBackground(null);
}
}
currentPlayer = 1;
winner = 0;
statusLabel.setText("当前玩家: " + currentPlayer);
}
public static void main(String[] args) {
new Gobang();
}
}
```
这个代码会创建一个15x15的棋盘,玩家点击棋盘上的格子可以下棋。当有一方获胜时,会显示胜利信息;玩家可以点击“重新开始”按钮重新开始游戏,也可以点击“悔棋”按钮悔棋。
相关推荐
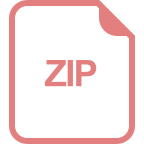
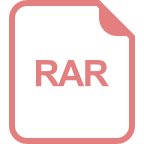














