用Java实现五子棋。要求:1.有图形用户界面。2.有开始游戏、悔棋、认输三个功能按钮。3.能够进行人人对战也能进行人机对战。4.能够再IDEA编译器上实现
时间: 2024-01-21 08:20:21 浏览: 179
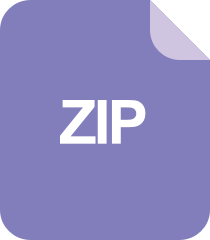
基于Java实现有界面的五子棋
好的,我可以给你提供一个简单的五子棋Java实现的代码,满足你的需求。首先,我们需要使用Java Swing库来创建图形用户界面。以下是示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class Gobang extends JFrame implements ActionListener, MouseListener{
private JPanel panel;
private JButton startBtn, regretBtn, giveUpBtn;
private JLabel messageLabel;
private int[][] chessBoard = new int[15][15];
private int currentPlayer = 1;
private boolean gameOver = false;
public Gobang(){
super("五子棋游戏");
setSize(500, 500);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
panel = new JPanel(new GridLayout(15, 15));
for(int i = 0; i < 15; i++){
for(int j = 0; j < 15; j++){
JButton button = new JButton();
button.setBackground(Color.WHITE);
button.setBorder(BorderFactory.createLineBorder(Color.BLACK));
button.addMouseListener(this);
panel.add(button);
}
}
startBtn = new JButton("开始游戏");
startBtn.addActionListener(this);
regretBtn = new JButton("悔棋");
regretBtn.addActionListener(this);
giveUpBtn = new JButton("认输");
giveUpBtn.addActionListener(this);
messageLabel = new JLabel("请点击开始游戏按钮", JLabel.CENTER);
JPanel buttonPanel = new JPanel(new FlowLayout());
buttonPanel.add(startBtn);
buttonPanel.add(regretBtn);
buttonPanel.add(giveUpBtn);
add(panel, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
add(messageLabel, BorderLayout.NORTH);
setVisible(true);
}
public void actionPerformed(ActionEvent e){
if(e.getSource() == startBtn){
startGame();
}else if(e.getSource() == regretBtn){
regret();
}else if(e.getSource() == giveUpBtn){
giveUp();
}
}
public void mouseClicked(MouseEvent e){
if(gameOver) return;
JButton button = (JButton)e.getSource();
int row = -1, col = -1;
Component[] components = panel.getComponents();
for(int i = 0; i < components.length; i++){
if(button.equals(components[i])){
row = i / 15;
col = i % 15;
break;
}
}
if(chessBoard[row][col] != 0) return;
chessBoard[row][col] = currentPlayer;
button.setBackground(currentPlayer == 1 ? Color.BLACK : Color.WHITE);
checkWin(row, col);
if(!gameOver){
currentPlayer = 3 - currentPlayer;
messageLabel.setText("当前玩家:" + (currentPlayer == 1 ? "黑方" : "白方"));
}
}
private void startGame(){
for(int i = 0; i < 15; i++){
for(int j = 0; j < 15; j++){
chessBoard[i][j] = 0;
Component[] components = panel.getComponents();
JButton button = (JButton)components[i * 15 + j];
button.setBackground(Color.WHITE);
}
}
currentPlayer = 1;
gameOver = false;
messageLabel.setText("当前玩家:黑方");
}
private void regret(){
if(gameOver) return;
int count = 0;
for(int i = 0; i < 15; i++){
for(int j = 0; j < 15; j++){
if(chessBoard[i][j] != 0) count++;
}
}
if(count <= 1) return;
int prevPlayer = 3 - currentPlayer;
for(int i = 14; i >= 0; i--){
for(int j = 14; j >= 0; j--){
if(chessBoard[i][j] == prevPlayer){
chessBoard[i][j] = 0;
Component[] components = panel.getComponents();
JButton button = (JButton)components[i * 15 + j];
button.setBackground(Color.WHITE);
messageLabel.setText("当前玩家:" + (currentPlayer == 1 ? "黑方" : "白方"));
gameOver = false;
return;
}
}
}
}
private void giveUp(){
if(gameOver) return;
int option = JOptionPane.showConfirmDialog(this, "确定要认输吗?", "提示", JOptionPane.YES_NO_OPTION);
if(option == JOptionPane.YES_OPTION){
gameOver = true;
messageLabel.setText(currentPlayer == 1 ? "黑方认输,白方胜利" : "白方认输,黑方胜利");
}
}
private void checkWin(int row, int col){
int count = 1;
int i, j;
i = row - 1; j = col;
while(i >= 0 && chessBoard[i][j] == currentPlayer){
count++;
i--;
}
i = row + 1; j = col;
while(i < 15 && chessBoard[i][j] == currentPlayer){
count++;
i++;
}
if(count >= 5){
gameOver = true;
messageLabel.setText(currentPlayer == 1 ? "黑方胜利" : "白方胜利");
return;
}
count = 1;
i = row; j = col - 1;
while(j >= 0 && chessBoard[i][j] == currentPlayer){
count++;
j--;
}
i = row; j = col + 1;
while(j < 15 && chessBoard[i][j] == currentPlayer){
count++;
j++;
}
if(count >= 5){
gameOver = true;
messageLabel.setText(currentPlayer == 1 ? "黑方胜利" : "白方胜利");
return;
}
count = 1;
i = row - 1; j = col - 1;
while(i >= 0 && j >= 0 && chessBoard[i][j] == currentPlayer){
count++;
i--;
j--;
}
i = row + 1; j = col + 1;
while(i < 15 && j < 15 && chessBoard[i][j] == currentPlayer){
count++;
i++;
j++;
}
if(count >= 5){
gameOver = true;
messageLabel.setText(currentPlayer == 1 ? "黑方胜利" : "白方胜利");
return;
}
count = 1;
i = row - 1; j = col + 1;
while(i >= 0 && j < 15 && chessBoard[i][j] == currentPlayer){
count++;
i--;
j++;
}
i = row + 1; j = col - 1;
while(i < 15 && j >= 0 && chessBoard[i][j] == currentPlayer){
count++;
i++;
j--;
}
if(count >= 5){
gameOver = true;
messageLabel.setText(currentPlayer == 1 ? "黑方胜利" : "白方胜利");
return;
}
int count2 = 0;
for(int k = 0; k < 15; k++){
if(chessBoard[k][col] == currentPlayer) count2++;
else count2 = 0;
if(count2 >= 5){
gameOver = true;
messageLabel.setText(currentPlayer == 1 ? "黑方胜利" : "白方胜利");
return;
}
}
count2 = 0;
for(int k = 0; k < 15; k++){
if(chessBoard[row][k] == currentPlayer) count2++;
else count2 = 0;
if(count2 >= 5){
gameOver = true;
messageLabel.setText(currentPlayer == 1 ? "黑方胜利" : "白方胜利");
return;
}
}
int count3 = 0;
for(int k = 0; k < 15; k++){
if(chessBoard[k][col] != 0) count3++;
}
for(int k = 0; k < 15; k++){
if(chessBoard[row][k] != 0) count3++;
}
if(row == col){
for(int k = 0; k < 15; k++){
if(chessBoard[k][k] != 0) count3++;
}
}
if(row == 14 - col){
for(int k = 0; k < 15; k++){
if(chessBoard[k][14 - k] != 0) count3++;
}
}
if(count3 == 225){
gameOver = true;
messageLabel.setText("平局");
}
}
public void mousePressed(MouseEvent e){}
public void mouseReleased(MouseEvent e){}
public void mouseEntered(MouseEvent e){}
public void mouseExited(MouseEvent e){}
public static void main(String[] args){
new Gobang();
}
}
```
上述代码使用了JPanel作为棋盘面板,使用JButton来表示棋子。通过给每个按钮添加MouseListener来监听鼠标点击事件,从而实现玩家下棋的功能。同时,我们还可以通过按钮来实现开始游戏、悔棋、认输等功能。
在代码中,我们使用了一个二维数组来表示棋盘的状态,其中0表示空位,1表示黑子,2表示白子。每次玩家下棋后,我们需要检查是否有五子连珠,如果有,则游戏结束,否则交换玩家。同时,我们还需要判断是否出现平局的情况。
以上就是一个简单的五子棋Java实现的示例代码,你可以在IDEA编译器上运行它。如果你对如何实现某个功能有疑问,可以在评论区留言。
阅读全文
相关推荐




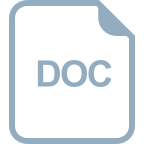
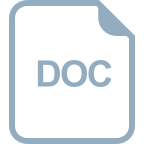
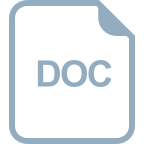
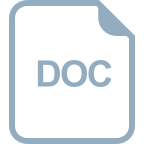
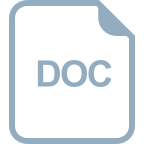
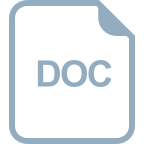
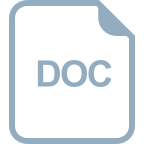




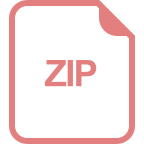
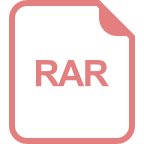