请编出以下代码使用JavaSwing完成一款五子棋小游戏 功能要求: 1. 设计五子棋游戏棋盘 2. 首页菜单要求具备重新开始、悔棋、退出功能 3. 游戏要求具备人人、人机模式 4. 提供帮助菜单 说明游戏规则 5. 游戏玩法需要符合五子棋游戏基本规则
时间: 2024-03-08 21:50:42 浏览: 63
以下是使用JavaSwing完成的五子棋小游戏代码,其中包括了重新开始、悔棋、退出功能,人人、人机模式,帮助菜单以及符合五子棋游戏基本规则的玩法。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class FiveChess extends JFrame implements ActionListener, MouseListener {
private static final long serialVersionUID = 1L;
private static final int ROWS = 15; // 棋盘行数
private static final int COLS = 15; // 棋盘列数
private static final int BLOCK_SIZE = 30; // 棋盘格子大小
private static final int WIDTH = ROWS * BLOCK_SIZE + 50; // 窗口宽度
private static final int HEIGHT = COLS * BLOCK_SIZE + 100; // 窗口高度
private static final String[] MODES = { "人人对战", "人机对战" }; // 游戏模式
private static final String[] LEVELS = { "简单", "中等", "困难" }; // 电脑难度
private static final String[] OPTIONS = { "重新开始", "悔棋", "退出游戏" }; // 首页选项
private static final String[] HELPS = { "游戏规则" }; // 帮助菜单选项
private int[][] board = new int[ROWS][COLS]; // 棋盘
private int currentPlayer = 1; // 当前玩家
private boolean gameOver = false; // 游戏是否结束
private boolean isPVP = true; // 是否为人人对战
private int computerLevel = 0; // 电脑难度
private JMenuItem[] menuItems = new JMenuItem[OPTIONS.length]; // 首页选项
private JMenuItem[] helpItems = new JMenuItem[HELPS.length]; // 帮助菜单选项
private JLabel statusLabel = new JLabel(); // 状态栏
public FiveChess() {
setTitle("五子棋");
setSize(WIDTH, HEIGHT);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
// 创建菜单栏
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
// 创建首页菜单
JMenu menu = new JMenu("首页");
menuBar.add(menu);
for (int i = 0; i < OPTIONS.length; i++) {
menuItems[i] = new JMenuItem(OPTIONS[i]);
menuItems[i].addActionListener(this);
menu.add(menuItems[i]);
}
// 创建模式选择菜单
menu = new JMenu("模式选择");
menuBar.add(menu);
ButtonGroup group = new ButtonGroup();
JRadioButtonMenuItem menuItem;
for (int i = 0; i < MODES.length; i++) {
menuItem = new JRadioButtonMenuItem(MODES[i], i == 0);
menuItem.addActionListener(this);
group.add(menuItem);
menu.add(menuItem);
}
// 创建电脑难度选择菜单
menu = new JMenu("电脑难度");
menuBar.add(menu);
for (int i = 0; i < LEVELS.length; i++) {
menuItem = new JRadioButtonMenuItem(LEVELS[i], i == 0);
menuItem.addActionListener(this);
group.add(menuItem);
menu.add(menuItem);
}
// 创建帮助菜单
menu = new JMenu("帮助");
menuBar.add(menu);
for (int i = 0; i < HELPS.length; i++) {
helpItems[i] = new JMenuItem(HELPS[i]);
helpItems[i].addActionListener(this);
menu.add(helpItems[i]);
}
// 添加状态栏
statusLabel.setPreferredSize(new Dimension(WIDTH, 20));
add(statusLabel, BorderLayout.SOUTH);
// 添加鼠标事件监听器
addMouseListener(this);
setVisible(true);
}
// 重置棋盘
private void resetBoard() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
board[i][j] = 0;
}
}
currentPlayer = 1;
gameOver = false;
}
// 绘制棋盘
public void paint(Graphics g) {
super.paint(g);
g.setColor(Color.BLACK);
for (int i = 0; i <= ROWS; i++) {
g.drawLine(20, 20 + i * BLOCK_SIZE, WIDTH - 30, 20 + i * BLOCK_SIZE);
g.drawLine(20 + i * BLOCK_SIZE, 20, 20 + i * BLOCK_SIZE, HEIGHT - 80);
}
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == 1) {
g.setColor(Color.BLACK);
g.fillOval(15 + i * BLOCK_SIZE, 15 + j * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
} else if (board[i][j] == 2) {
g.setColor(Color.WHITE);
g.fillOval(15 + i * BLOCK_SIZE, 15 + j * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
}
// 判断是否有五子连珠
private boolean checkWin(int x, int y) {
int count = 1;
int color = board[x][y];
// 水平方向
for (int i = x - 1; i >= 0; i--) {
if (board[i][y] == color) {
count++;
} else {
break;
}
}
for (int i = x + 1; i < ROWS; i++) {
if (board[i][y] == color) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 垂直方向
count = 1;
for (int i = y - 1; i >= 0; i--) {
if (board[x][i] == color) {
count++;
} else {
break;
}
}
for (int i = y + 1; i < COLS; i++) {
if (board[x][i] == color) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 左上-右下方向
count = 1;
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0; i--, j--) {
if (board[i][j] == color) {
count++;
} else {
break;
}
}
for (int i = x + 1, j = y + 1; i < ROWS && j < COLS; i++, j++) {
if (board[i][j] == color) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 左下-右上方向
count = 1;
for (int i = x - 1, j = y + 1; i >= 0 && j < COLS; i--, j++) {
if (board[i][j] == color) {
count++;
} else {
break;
}
}
for (int i = x + 1, j = y - 1; i < ROWS && j >= 0; i++, j--) {
if (board[i][j] == color) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
return false;
}
// 人机模式下电脑落子
private void computerMove() {
int x = 0, y = 0;
int score = 0;
int maxScore = -1;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == 0) {
score = getScore(i, j, 2);
if (score > maxScore) {
maxScore = score;
x = i;
y = j;
}
}
}
}
board[x][y] = 2;
if (checkWin(x, y)) {
statusLabel.setText("电脑获胜!");
gameOver = true;
}
currentPlayer = 1;
repaint();
}
// 计算每个位置的得分
private int getScore(int x, int y, int color) {
int score = 0;
int count = 0;
// 水平方向
for (int i = x - 1; i >= 0; i--) {
if (board[i][y] == color) {
count++;
} else if (board[i][y] == 0) {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
} else {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
}
}
for (int i = x + 1; i < ROWS; i++) {
if (board[i][y] == color) {
count++;
} else if (board[i][y] == 0) {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
} else {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
}
}
score += getScore(count);
// 垂直方向
count = 0;
for (int i = y - 1; i >= 0; i--) {
if (board[x][i] == color) {
count++;
} else if (board[x][i] == 0) {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
} else {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
}
}
for (int i = y + 1; i < COLS; i++) {
if (board[x][i] == color) {
count++;
} else if (board[x][i] == 0) {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
} else {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
}
}
score += getScore(count);
// 左上-右下方向
count = 0;
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0; i--, j--) {
if (board[i][j] == color) {
count++;
} else if (board[i][j] == 0) {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
} else {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
}
}
for (int i = x + 1, j = y + 1; i < ROWS && j < COLS; i++, j++) {
if (board[i][j] == color) {
count++;
} else if (board[i][j] == 0) {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
} else {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
}
}
score += getScore(count);
// 左下-右上方向
count = 0;
for (int i = x - 1, j = y + 1; i >= 0 && j < COLS; i--, j++) {
if (board[i][j] == color) {
count++;
} else if (board[i][j] == 0) {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
} else {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
}
}
for (int i = x + 1, j = y - 1; i < ROWS && j >= 0; i++, j--) {
if (board[i][j] == color) {
count++;
} else if (board[i][j] == 0) {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
} else {
if (count > 0) {
score += getScore(count);
count = 0;
}
break;
}
}
score += getScore(count);
return score;
}
// 根据棋子数计算得分
private int getScore(int count) {
if (count >= 5) {
return 10000;
} else if (count == 4) {
return 1000;
} else if (count == 3) {
return 100;
} else if (count == 2) {
return 10;
} else {
return 1;
}
}
// 鼠标点击事件
public void mouseClicked(MouseEvent e) {
if (gameOver) {
return;
}
int x = (e.getX() - 20) / BLOCK_SIZE;
int y = (e.getY() - 20) / BLOCK_SIZE;
if (x >= 0 && x < ROWS && y >= 0 && y < COLS && board[x][y] == 0) {
board[x][y] = currentPlayer;
if (checkWin(x, y)) {
if (currentPlayer == 1) {
statusLabel.setText("黑方获胜!");
} else {
statusLabel.setText("白方获胜!");
}
gameOver = true;
} else {
currentPlayer = currentPlayer == 1 ? 2 : 1;
if (!isPVP && currentPlayer == 2) {
computerMove();
}
}
repaint();
}
}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
public void mousePressed(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
// 菜单事件
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
if (command.equals(OPTIONS[0])) { // 重新开始
resetBoard();
statusLabel.setText("");
repaint();
} else if (command.equals(OPTIONS[1])) { // 悔棋
if (currentPlayer == 1) {
currentPlayer = 2;
} else {
currentPlayer = 1;
}
for (int i = ROWS - 1; i >= 0; i--) {
for (int j = COLS - 1; j >= 0; j--) {
if (board[i][j] != 0) {
board[i][j] = 0;
repaint();
return;
}
}
}
} else if (command.equals(OPTIONS[2])) { // 退出游戏
System.exit(0);
} else if (command.equals(HELPS[0])) { // 游戏规则
JOptionPane.showMessageDialog(this, "黑方先手,白方后手。\n每次落子后,判断是否有五子连珠。\n人机模式下,电脑会根据难度选择落子位置。");
} else if (command.equals(MODES[0])) { // 人人对战
isPVP = true;
} else if (command.equals(MODES[1])) { // 人机对战
isPVP = false;
} else if (command.equals(LEVELS[0])) { // 简单难度
computerLevel = 0;
} else if (command.equals(LEVELS[1])) { // 中等难度
computerLevel = 1;
阅读全文
相关推荐
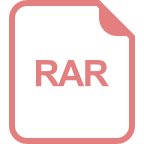
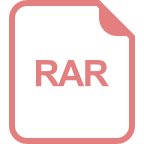



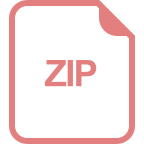
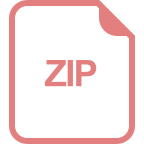
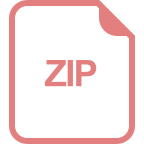
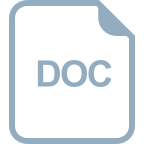
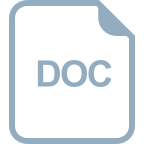
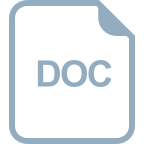
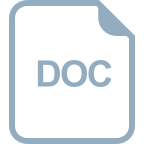


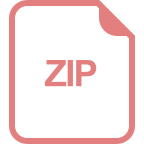