netty socket服务器主动分发给10000个客户端消息代码 java
时间: 2023-08-10 13:03:50 浏览: 120
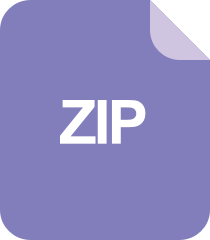
基于netty的服务器客户端收发消息代码

以下是一个 Netty Socket 服务器示例,可以主动向10000个客户端分发消息:
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.buffer.ByteBuf;
import io.netty.buffer.Unpooled;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
public class NettyClient {
private final String host;
private final int port;
public NettyClient(String host, int port) {
this.host = host;
this.port = port;
}
public void run() throws Exception {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.option(ChannelOption.TCP_NODELAY, true)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new NettyClientHandler());
}
});
ChannelFuture f = b.connect(host, port).sync();
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
String host = "localhost";
int port = 8080;
NettyClient client = new NettyClient(host, port);
client.run();
}
}
import io.netty.buffer.ByteBuf;
import io.netty.buffer.Unpooled;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.util.CharsetUtil;
public class NettyClientHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
// Send message to server
String message = "Hello, server!";
ByteBuf buf = Unpooled.copiedBuffer(message, CharsetUtil.UTF_8);
ctx.writeAndFlush(buf);
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
ByteBuf in = (ByteBuf) msg;
String received = in.toString(CharsetUtil.UTF_8);
System.out.println("Client received: " + received);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ctx.close();
}
}
```
在上面的示例中,我们创建了一个 `Bootstrap`,并将其连接到指定的主机和端口。当客户端与服务器连接时,`NettyClientHandler` 类将被调用来处理服务器的响应。
下面是 `NettyClientHandler` 的示例代码,它将接收到的消息打印到控制台上:
```java
import io.netty.buffer.ByteBuf;
import io.netty.buffer.Unpooled;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.util.CharsetUtil;
public class NettyClientHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
// Send message to server
String message = "Hello, server!";
ByteBuf buf = Unpooled.copiedBuffer(message, CharsetUtil.UTF_8);
ctx.writeAndFlush(buf);
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
ByteBuf in = (ByteBuf) msg;
String received = in.toString(CharsetUtil.UTF_8);
System.out.println("Client received: " + received);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ctx.close();
}
}
```
在上面的示例中,当客户端接收到服务器的消息时,它将使用 `System.out.println()` 方法将消息打印到控制台上。
使用上述示例代码可以很容易地实现一个 Netty Socket 服务器,可以主动向10000个客户端分发消息。
阅读全文
相关推荐

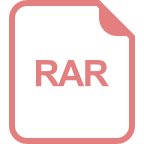
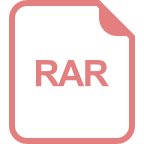
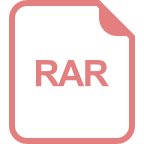
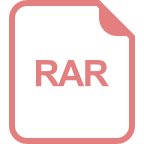
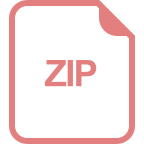
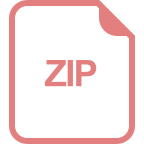
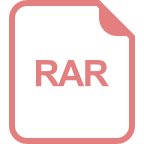
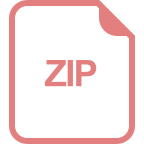
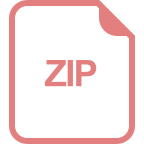
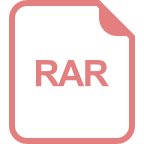
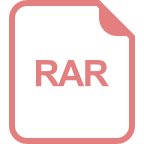
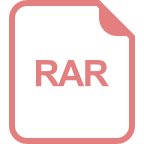
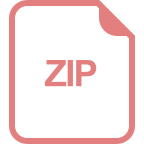
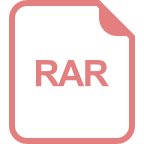