#include <math.h> #include <stdio.h> #include <stdlib.h> double fun(int n) { double s = 0.0; int i; /**********Program**********/ int y; for (i=1;i<n;i++) { if (i%5==0 && i%11==0) { s=s+i; } } s=s^0.5; return s; /********** End **********/ } int main() { FILE *wf; system("CLS"); printf("s=%f\n", fun(1000)); wf=fopen("out.txt", "w"); fprintf(wf, "%f", fun(1000)); fclose(wf); }
时间: 2023-06-29 07:18:16 浏览: 155
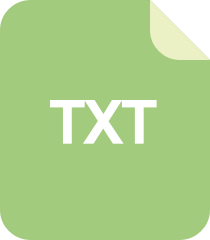
#include <stdio.h>

这段代码中定义了一个名为fun()的函数,该函数使用一个for循环来遍历从1到n-1的整数,判断是否同时能被5和11整除,如果可以则将其加入到s中。最后,将s的平方根返回。
在主函数中,调用fun()函数来计算从1到999中同时能被5和11整除的数的平方根,并将结果输出到屏幕和out.txt文件中。
需要注意的是,代码中对double类型的变量进行了异或运算(s=s^0.5;),这是不正确的,应该使用sqrt()函数来计算平方根,如下所示:
```c
#include <math.h>
#include <stdio.h>
#include <stdlib.h>
double fun(int n) {
double s = 0.0;
int i;
for (i = 1; i < n; i++) {
if (i % 5 == 0 && i % 11 == 0) {
s += i;
}
}
s = sqrt(s);
return s;
}
int main() {
FILE *wf;
system("CLS");
printf("s=%f\n", fun(1000));
wf = fopen("out.txt", "w");
fprintf(wf, "%f", fun(1000));
fclose(wf);
return 0;
}
```
在上面的修改后的代码中,我们使用了math.h头文件中的sqrt()函数来计算s的平方根,而不是使用异或运算。
阅读全文
相关推荐
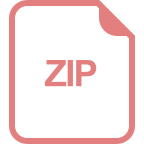
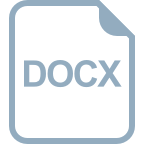










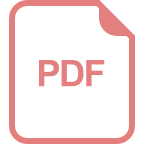




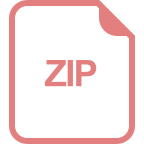