建立一个简单的运动会管理系统,包括田赛和径赛的管理。需要完成如下功能: 1、建立一个基类SportEvent,它的属性有比赛名称name、比赛地点location,参赛的运动员athlete;还要给SportEvent类定义合适的成员函数,其中包括虚函数show_info (),用于输出比赛项目的基本信息。 2、建立一个田赛类FieldEvent和一个径赛类TrackEvent,它们均由SportEvent类公有继承而来。 3、田赛类FieldEvent有新增数据成员:运动员跳的距离distance,给田赛类重定义show_info ()函数以输出其相关信息。田赛类还应该具有修改距离等功能和比较运动员的成绩。 4、径赛类TrackEvent有新增数据成员:运动员跑的时间time,给径赛类重定义show_info ()函数以输出其相关信息。径赛类还应该具有修改时间和比较运动员的成绩等功能。 5、重载>>,实现用cin为田赛类和径赛类的对象取得值。 6、为每个类写构造函数。田赛对象和径赛对象的信息都能够保存到文件。 7、编写main()主函数,测试上述功能。
时间: 2023-06-16 11:07:04 浏览: 130
以下是运动会管理系统的代码实现,可以根据需求进行调整:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
class SportEvent {
protected:
string name;
string location;
vector<string> athletes;
public:
SportEvent(string n, string l) : name(n), location(l) {}
virtual void show_info() {
cout << "比赛名称:" << name << endl;
cout << "比赛地点:" << location << endl;
cout << "参赛运动员:" << endl;
for (auto a : athletes) {
cout << a << endl;
}
}
void add_athlete(string athlete) {
athletes.push_back(athlete);
}
void modify_location(string l) {
location = l;
}
virtual ~SportEvent() {}
};
class FieldEvent : public SportEvent {
private:
double distance;
public:
FieldEvent(string n, string l, double d) : SportEvent(n, l), distance(d) {}
void show_info() {
SportEvent::show_info();
cout << "运动员跳的距离:" << distance << endl;
}
void modify_distance(double d) {
distance = d;
}
int compare(FieldEvent& other) {
if (distance > other.distance) {
return 1;
} else if (distance < other.distance) {
return -1;
} else {
return 0;
}
}
};
class TrackEvent : public SportEvent {
private:
double time;
public:
TrackEvent(string n, string l, double t) : SportEvent(n, l), time(t) {}
void show_info() {
SportEvent::show_info();
cout << "运动员跑的时间:" << time << endl;
}
void modify_time(double t) {
time = t;
}
int compare(TrackEvent& other) {
if (time < other.time) {
return 1;
} else if (time > other.time) {
return -1;
} else {
return 0;
}
}
};
istream& operator>>(istream& input, FieldEvent& event) {
input >> event.name >> event.location >> event.distance;
return input;
}
istream& operator>>(istream& input, TrackEvent& event) {
input >> event.name >> event.location >> event.time;
return input;
}
void save_to_file(SportEvent& event) {
ofstream fout(event.name + ".txt");
fout << event.name << endl;
fout << event.location << endl;
for (auto a : event.athletes) {
fout << a << endl;
}
fout.close();
}
int main() {
FieldEvent field("跳远比赛", "田径场", 7.85);
field.add_athlete("张三");
field.add_athlete("李四");
field.show_info();
field.modify_distance(8.0);
field.show_info();
FieldEvent field2("三级跳远比赛", "田径场", 15.2);
field2.add_athlete("王五");
field2.add_athlete("赵六");
field2.show_info();
cout << "比较结果:" << field.compare(field2) << endl;
TrackEvent track("100米赛跑", "田径场", 9.88);
track.add_athlete("小明");
track.add_athlete("小红");
track.show_info();
track.modify_time(9.56);
track.show_info();
TrackEvent track2("200米赛跑", "田径场", 19.77);
track2.add_athlete("小刚");
track2.add_athlete("小芳");
track2.show_info();
cout << "比较结果:" << track.compare(track2) << endl;
cin >> field >> track;
field.show_info();
track.show_info();
save_to_file(field);
save_to_file(track);
return 0;
}
```
在上述实现中,使用了类的继承、虚函数、重载运算符等基本的面向对象编程的概念。可以通过调用各个类的函数来实现对比赛项目信息的管理,包括添加运动员、修改比赛地点、修改成绩等操作,并且可以通过文件保存来保存比赛信息。
阅读全文
相关推荐

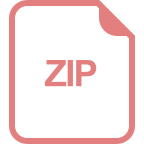
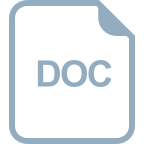
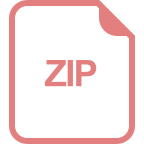
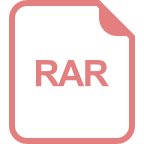
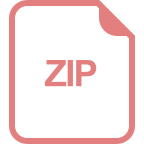
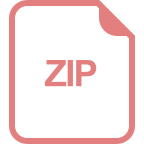
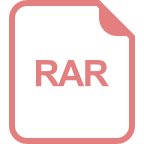
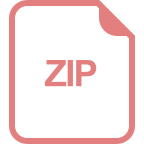
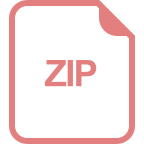
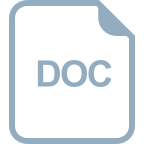


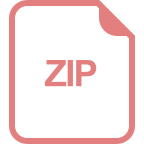