用C++写一个设备管理系统的增删改查
时间: 2023-10-05 12:12:46 浏览: 71
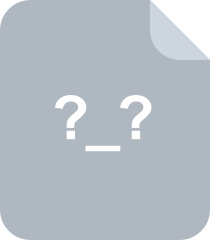
C语言,设备管理系统,用win32写的界面,增删改查基本功能都有,
好的,以下是一个简单的设备管理系统的增删改查的 C++ 代码示例:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
struct Device {
string name;
int id;
int quantity;
};
vector<Device> devices;
void add_device() {
Device device;
cout << "请输入设备名称:";
cin >> device.name;
cout << "请输入设备编号:";
cin >> device.id;
cout << "请输入设备数量:";
cin >> device.quantity;
devices.push_back(device);
cout << "设备信息已添加!" << endl;
}
void delete_device() {
int id;
cout << "请输入要删除的设备编号:";
cin >> id;
for (auto it = devices.begin(); it != devices.end(); ++it) {
if (it->id == id) {
devices.erase(it);
cout << "设备信息已删除!" << endl;
return;
}
}
cout << "未找到该设备编号的设备信息!" << endl;
}
void update_device() {
int id;
cout << "请输入要修改的设备编号:";
cin >> id;
for (auto it = devices.begin(); it != devices.end(); ++it) {
if (it->id == id) {
cout << "请输入修改后的设备名称:";
cin >> it->name;
cout << "请输入修改后的设备数量:";
cin >> it->quantity;
cout << "设备信息已修改!" << endl;
return;
}
}
cout << "未找到该设备编号的设备信息!" << endl;
}
void search_device() {
int id;
cout << "请输入要查询的设备编号:";
cin >> id;
for (auto it = devices.begin(); it != devices.end(); ++it) {
if (it->id == id) {
cout << "设备名称:" << it->name << endl;
cout << "设备数量:" << it->quantity << endl;
return;
}
}
cout << "未找到该设备编号的设备信息!" << endl;
}
void print_menu() {
cout << "1. 添加设备信息" << endl;
cout << "2. 删除设备信息" << endl;
cout << "3. 修改设备信息" << endl;
cout << "4. 查询设备信息" << endl;
cout << "5. 退出系统" << endl;
}
int main() {
while (true) {
print_menu();
int choice;
cout << "请输入您的选择:";
cin >> choice;
switch (choice) {
case 1:
add_device();
break;
case 2:
delete_device();
break;
case 3:
update_device();
break;
case 4:
search_device();
break;
case 5:
cout << "欢迎下次再来!" << endl;
return 0;
default:
cout << "输入有误,请重新输入!" << endl;
}
}
}
```
这个程序使用了一个 `vector` 存储所有的设备信息,通过循环遍历容器来实现增删改查功能。每个操作都有对应的函数,用户通过输入数字选择要执行的操作。
阅读全文
相关推荐
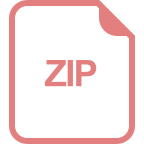
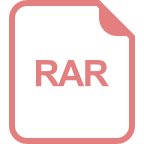
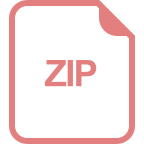
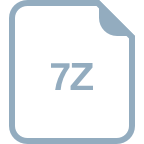
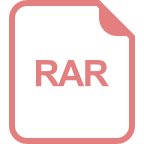
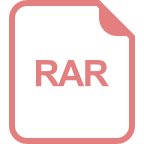
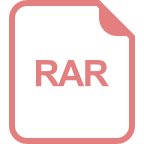
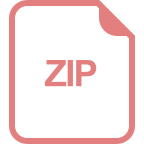
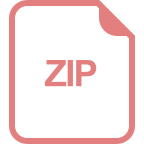
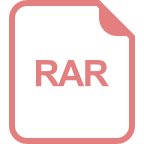
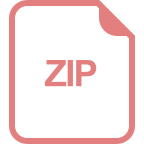
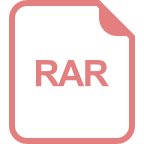
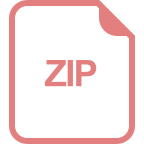