2.自已拟定一个解决实际简单题目,分析并抽象为一个类,然后定义对象测试(类的定义放在头文件中,测试程序用exp_105.cpp保存)
时间: 2024-02-13 14:01:32 浏览: 54
假设我要设计一个简单的学生类,用于描述一个学生的姓名、年龄、性别、学号和成绩等属性,以及计算学生平均成绩的功能。
下面是学生类的头文件 Student.h:
```c++
#ifndef STUDENT_H
#define STUDENT_H
#include <string>
#include <vector>
class Student {
private:
std::string name; // 姓名
int age; // 年龄
std::string gender; // 性别
std::string id; // 学号
std::vector<double> scores; // 成绩列表
public:
Student(std::string name, int age, std::string gender, std::string id); // 构造函数
~Student(); // 析构函数
void addScore(double score); // 添加成绩
double getAverageScore(); // 计算平均成绩
std::string getName(); // 获取姓名
int getAge(); // 获取年龄
std::string getGender(); // 获取性别
std::string getId(); // 获取学号
};
#endif
```
下面是学生类的实现文件 Student.cpp:
```c++
#include "Student.h"
Student::Student(std::string name, int age, std::string gender, std::string id) {
this->name = name;
this->age = age;
this->gender = gender;
this->id = id;
}
Student::~Student() {}
void Student::addScore(double score) {
this->scores.push_back(score);
}
double Student::getAverageScore() {
double sum = 0;
for (int i = 0; i < this->scores.size(); i++) {
sum += this->scores[i];
}
return sum / this->scores.size();
}
std::string Student::getName() {
return this->name;
}
int Student::getAge() {
return this->age;
}
std::string Student::getGender() {
return this->gender;
}
std::string Student::getId() {
return this->id;
}
```
下面是测试程序 exp_105.cpp:
```c++
#include <iostream>
#include "Student.h"
using namespace std;
int main() {
// 实例化一个学生对象
Student stu("Alice", 18, "female", "10001");
// 添加成绩
stu.addScore(80);
stu.addScore(90);
stu.addScore(85);
// 获取学生的姓名、年龄、性别和学号
cout << "Name: " << stu.getName() << endl;
cout << "Age: " << stu.getAge() << endl;
cout << "Gender: " << stu.getGender() << endl;
cout << "ID: " << stu.getId() << endl;
// 计算学生的平均成绩
double avgScore = stu.getAverageScore();
cout << "Average score: " << avgScore << endl;
return 0;
}
```
该测试程序实例化了一个学生对象,添加了几门课程的成绩,获取了学生的姓名、年龄、性别和学号,并计算了学生的平均成绩。
阅读全文
相关推荐
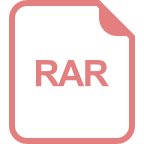
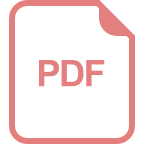
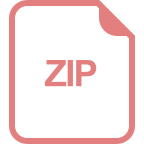














