用单片机代码写出简易信号分析仪
时间: 2024-02-28 11:24:49 浏览: 14
要实现简易信号分析仪,至少需要以下硬件和软件:
硬件:
1. 单片机开发板(推荐STM32F103C8T6)
2. LCD屏幕
3. AD转换器(ADC)模块
4. 信号源(可以是任何可以输出信号的设备,如手机、电脑等)
软件:
1. Keil或其他单片机开发软件
2. ST-Link或其他下载器
下面是一个简单的单片机代码,用于实现一个基本的信号分析仪。
```c
#include "stm32f10x.h"
#include "stdio.h"
#define ADC1_DR_Address ((uint32_t)0x4001244C) //ADC1 data register address
#define LCD_RS GPIO_Pin_0 //LCD control pins
#define LCD_RW GPIO_Pin_1
#define LCD_E GPIO_Pin_2
#define LCD_D4 GPIO_Pin_4 //LCD data pins
#define LCD_D5 GPIO_Pin_5
#define LCD_D6 GPIO_Pin_6
#define LCD_D7 GPIO_Pin_7
void init_ADC(void);
u16 read_ADC(u8 channel);
void init_GPIO(void);
void init_LCD(void);
void LCD_send_byte(u8 data);
void LCD_send_command(u8 command);
void LCD_send_data(u8 data);
void LCD_gotoxy(u8 x, u8 y);
void LCD_print(char *string);
int main(void)
{
u16 adc_value; //declare variable to store ADC value
char buffer[16]; //declare buffer for storing string to display on LCD
init_GPIO(); //initialize GPIO pins
init_LCD(); //initialize LCD
init_ADC(); //initialize ADC
while(1)
{
adc_value = read_ADC(0); //read ADC value from channel 0
sprintf(buffer, "ADC Value: %04d", adc_value); //convert ADC value to string
LCD_gotoxy(0, 0); //set LCD cursor to (0,0)
LCD_print(buffer); //print ADC value on LCD
}
}
void init_ADC(void)
{
ADC_InitTypeDef ADC_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1 | RCC_APB2Periph_GPIOA, ENABLE);
/* Configure PA0 (ADC Channel0) as analog input -------------------------*/
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN;
GPIO_Init(GPIOA, &GPIO_InitStructure);
/* ADC1 Configuration ------------------------------------------------------*/
ADC_DeInit(ADC1);
ADC_InitStructure.ADC_Mode = ADC_Mode_Independent;
ADC_InitStructure.ADC_ScanConvMode = DISABLE;
ADC_InitStructure.ADC_ContinuousConvMode = ENABLE;
ADC_InitStructure.ADC_ExternalTrigConv = ADC_ExternalTrigConv_None;
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStructure.ADC_NbrOfChannel = 1;
ADC_Init(ADC1, &ADC_InitStructure);
/* ADC1 regular channel0 configuration -----------------------------------*/
ADC_RegularChannelConfig(ADC1, ADC_Channel_0, 1, ADC_SampleTime_55Cycles5);
/* Enable ADC1 */
ADC_Cmd(ADC1, ENABLE);
/* Enable ADC1 reset calibration register */
ADC_ResetCalibration(ADC1);
/* Check the end of ADC1 reset calibration register */
while(ADC_GetResetCalibrationStatus(ADC1));
/* Start ADC1 calibration */
ADC_StartCalibration(ADC1);
/* Check the end of ADC1 calibration */
while(ADC_GetCalibrationStatus(ADC1));
}
u16 read_ADC(u8 channel)
{
ADC_RegularChannelConfig(ADC1, channel, 1, ADC_SampleTime_55Cycles5); //configure ADC channel
ADC_SoftwareStartConvCmd(ADC1, ENABLE); //start ADC conversion
while(!ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC)); //wait for conversion to complete
return ADC_GetConversionValue(ADC1); //return ADC value
}
void init_GPIO(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = LCD_RS | LCD_RW | LCD_E | LCD_D4 | LCD_D5 | LCD_D6 | LCD_D7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_2MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
void init_LCD(void)
{
LCD_send_command(0x02); //initialize LCD in 4-bit mode
LCD_send_command(0x28); //2 lines, 5x8 character font
LCD_send_command(0x0C); //display on, cursor off, blink off
LCD_send_command(0x06); //increment cursor
LCD_send_command(0x01); //clear display
delay_ms(2); //wait for LCD to clear
}
void LCD_send_byte(u8 data)
{
GPIO_Write(GPIOB, (GPIO_ReadOutputData(GPIOB) & 0xFF00) | data); //write data to GPIO pins
GPIO_SetBits(GPIOB, LCD_E); //set enable pin
delay_us(1); //wait for enable pulse to complete
GPIO_ResetBits(GPIOB, LCD_E); //clear enable pin
}
void LCD_send_command(u8 command)
{
GPIO_ResetBits(GPIOB, LCD_RS | LCD_RW); //set control pins to command mode
LCD_send_byte((command >> 4) & 0x0F); //send high nibble
LCD_send_byte(command & 0x0F); //send low nibble
}
void LCD_send_data(u8 data)
{
GPIO_SetBits(GPIOB, LCD_RS); //set RS pin to data mode
GPIO_ResetBits(GPIOB, LCD_RW); //set RW pin to write mode
LCD_send_byte((data >> 4) & 0x0F); //send high nibble
LCD_send_byte(data & 0x0F); //send low nibble
}
void LCD_gotoxy(u8 x, u8 y)
{
u8 address = (0x40 * y) + x; //calculate DDRAM address
LCD_send_command(0x80 | address); //set DDRAM address
}
void LCD_print(char *string)
{
while(*string) //loop until null terminator is reached
{
LCD_send_data(*string++); //send character to LCD
}
}
void delay_ms(u16 ms)
{
u16 i, j;
for(i = 0; i < ms; i++)
{
for(j = 0; j < 6000; j++);
}
}
void delay_us(u16 us)
{
u16 i, j;
for(i = 0; i < us; i++)
{
for(j = 0; j < 10; j++);
}
}
```
这个代码实现了以下功能:
1. 初始化GPIO和LCD,使它们可以与单片机通信。
2. 初始化ADC模块,使它可以读取模拟信号。
3. 循环读取ADC值,并将其显示在LCD上。
请注意,这只是一个基本的示例。为了实现更高级的信号分析功能,您需要更多的硬件和更复杂的代码。
相关推荐
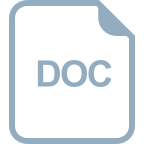
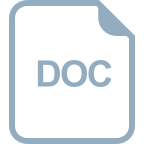














