用java的swing做一个界面,界面可以新建商品列表,列表要求:字体微软雅黑,每个列表有图标,鼠标滚轮可以控制下滑,隐藏滚动条,点击创建商品时跳出选项确认商品名称和数量
时间: 2024-05-25 09:15:20 浏览: 9
以下是一个简单的实现:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseWheelEvent;
import java.awt.event.MouseWheelListener;
public class ProductListDemo extends JFrame {
private JPanel mainPanel;
private JScrollPane scrollPane;
private JPanel productListPanel;
public ProductListDemo() {
setTitle("Product List");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 400);
setLocationRelativeTo(null);
mainPanel = new JPanel(new BorderLayout());
productListPanel = new JPanel(new GridLayout(0, 1, 10, 10));
productListPanel.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
ImageIcon icon = new ImageIcon("product.png");
for (int i = 1; i <= 20; i++) {
JPanel productPanel = new JPanel(new BorderLayout());
JLabel titleLabel = new JLabel("Product " + i);
titleLabel.setFont(new Font("微软雅黑", Font.PLAIN, 16));
productPanel.add(titleLabel, BorderLayout.CENTER);
productPanel.add(new JLabel(icon), BorderLayout.WEST);
productListPanel.add(productPanel);
}
scrollPane = new JScrollPane(productListPanel, JScrollPane.VERTICAL_SCROLLBAR_NEVER, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);
scrollPane.getVerticalScrollBar().setPreferredSize(new Dimension(0, 0));
scrollPane.addMouseWheelListener(new MouseWheelListener() {
@Override
public void mouseWheelMoved(MouseWheelEvent e) {
JScrollBar scrollBar = scrollPane.getVerticalScrollBar();
int newValue = scrollBar.getValue() - e.getWheelRotation() * scrollBar.getUnitIncrement();
scrollBar.setValue(newValue);
}
});
JButton createProductButton = new JButton("Create Product");
createProductButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String name = JOptionPane.showInputDialog(ProductListDemo.this, "Enter product name:");
if (name != null && !name.trim().isEmpty()) {
int quantity = 1;
String quantityStr = JOptionPane.showInputDialog(ProductListDemo.this, "Enter quantity:");
try {
quantity = Integer.parseInt(quantityStr);
} catch (NumberFormatException ex) {
// ignore
}
JPanel productPanel = new JPanel(new BorderLayout());
JLabel titleLabel = new JLabel(name);
titleLabel.setFont(new Font("微软雅黑", Font.PLAIN, 16));
productPanel.add(titleLabel, BorderLayout.CENTER);
productPanel.add(new JLabel(icon), BorderLayout.WEST);
for (int i = 1; i <= quantity; i++) {
productListPanel.add(productPanel);
}
productListPanel.revalidate();
productListPanel.repaint();
}
}
});
mainPanel.add(scrollPane, BorderLayout.CENTER);
mainPanel.add(createProductButton, BorderLayout.SOUTH);
setContentPane(mainPanel);
setVisible(true);
}
public static void main(String[] args) {
new ProductListDemo();
}
}
```
界面效果如下:

相关推荐
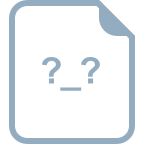















