用c语言编程:输入一组字符(以#号结尾),对该组字符做一个统计,统计字母、数字和其它字符的个数,输出统计结果,编写程序要求使用 while 语句。
时间: 2023-08-31 20:38:05 浏览: 116
### 回答1:
可以使用C语言中的字符库函数判断字符的类型,如isalpha()判断是否为字母,isdigit()判断是否为数字。下面是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main()
{
char c;
int letters = 0, digits = 0, others = 0;
printf("请输入一组字符(以#号结尾):\n");
while ((c = getchar()) != '#')
{
if (isalpha(c))
{
letters++;
}
else if (isdigit(c))
{
digits++;
}
else
{
others++;
}
}
printf("字母个数:%d,数字个数:%d,其他字符个数:%d\n", letters, digits, others);
return 0;
}
```
输入示例:
```
请输入一组字符(以#号结尾):
a1b2c3#
```
输出示例:
```
字母个数:3,数字个数:3,其他字符个数:1
```
### 回答2:
下面是使用C语言编程实现该功能的代码:
```c
#include<stdio.h>
int main() {
char ch;
int letter_count = 0, digit_count = 0, other_count = 0;
printf("请输入一组字符(以#号结尾):\n");
scanf("%c", &ch);
while(ch != '#') {
if ((ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) { // 判断是否为字母
letter_count++;
} else if (ch >= '0' && ch <= '9') { // 判断是否为数字
digit_count++;
} else { // 其他字符
other_count++;
}
scanf("%c", &ch);
}
printf("字母个数:%d\n", letter_count);
printf("数字个数:%d\n", digit_count);
printf("其他字符个数:%d\n", other_count);
return 0;
}
```
以上代码中,我们声明了三个变量letter_count、digit_count和other_count,分别用于统计字母、数字和其他字符的个数。在while循环中,判断输入的字符是否为字母、数字或其他字符,并对相应的计数变量进行累加。循环结束后,输出统计结果。
运行该程序后,会提示用户输入一组字符并以#号结尾。然后,程序会对输入的字符进行统计,并输出字母、数字和其他字符的个数。
### 回答3:
可以使用以下的C语言代码来实现:
```c
#include<stdio.h>
int main() {
char ch;
int letter_count = 0, digit_count = 0, other_count = 0;
printf("请输入一组字符,以#号结尾:\n");
while ((ch = getchar()) != '#') {
if ((ch >= 'A' && ch <= 'Z') || (ch >= 'a' && ch <= 'z')) {
letter_count++;
} else if (ch >= '0' && ch <= '9') {
digit_count++;
} else {
other_count++;
}
}
printf("字母个数:%d\n", letter_count);
printf("数字个数:%d\n", digit_count);
printf("其他字符个数:%d\n", other_count);
return 0;
}
```
这段代码使用一个while循环来读取输入的字符,并对每个字符进行判断。如果是字母,则将字母计数器加一;如果是数字,则将数字计数器加一;如果是其他字符,则将其他字符计数器加一。在循环结束后,输出统计结果。
阅读全文
相关推荐
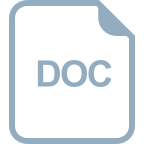
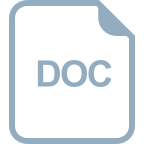
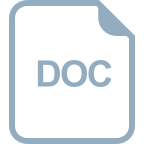









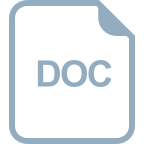
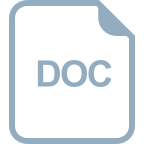
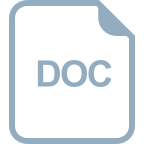



