编写c++算法,实现图的深度优先遍历。
时间: 2023-10-30 13:19:09 浏览: 84
好的,以下是一个简单的C++算法,用于实现图的深度优先遍历(DFS):
```c++
#include <iostream>
#include <vector>
#include <stack>
using namespace std;
// 定义图的数据结构
class Graph {
private:
int V; // 顶点数量
vector<int>* adj; // 邻接表
public:
Graph(int V) {
this->V = V;
adj = new vector<int>[V];
}
void addEdge(int v, int w) {
adj[v].push_back(w); // 将w添加到v的邻接表中
}
// DFS遍历
void DFS(int s) {
vector<bool> visited(V, false); // 定义visited数组表示顶点是否被访问过
stack<int> stack; // 定义一个栈用于存储顶点
// 将起始顶点s入栈,并将其标记为已访问
stack.push(s);
visited[s] = true;
while (!stack.empty()) {
// 弹出栈顶元素
int currentVertex = stack.top();
stack.pop();
cout << currentVertex << " ";
// 遍历当前顶点的邻居
for (auto i = adj[currentVertex].begin(); i != adj[currentVertex].end(); ++i) {
// 如果邻居未被访问过,则将其入栈,并将其标记为已访问
if (!visited[*i]) {
stack.push(*i);
visited[*i] = true;
}
}
}
}
};
int main() {
// 创建图并添加边
Graph g(5);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 3);
g.addEdge(2, 3);
g.addEdge(3, 4);
cout << "DFS遍历结果: ";
g.DFS(0);
return 0;
}
```
在上面的代码中,我们首先定义了一个图的数据结构,并实现了一个`DFS`函数来进行深度优先遍历。访问顶点时,我们将其加入栈中,并在访问邻居时,将其加入栈中。这样就可以保证我们按照深度优先的顺序遍历整个图。
阅读全文
相关推荐
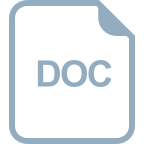
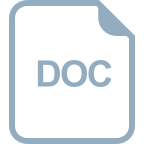
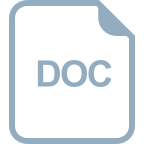

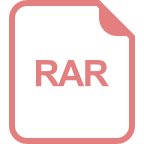
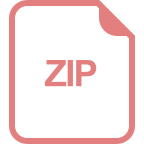
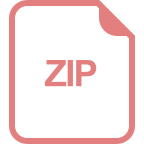
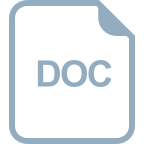
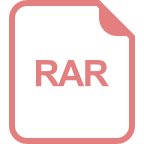
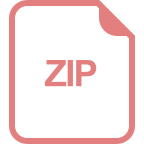
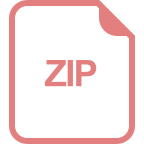
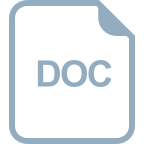





