NMPC控制器的simulink实例及其代码
时间: 2023-10-04 17:12:15 浏览: 203
以下是一个基于Simulink的NMPC控制器实例,用于控制一个非线性水箱系统:
1. 建立非线性水箱系统的动态模型,包括水箱的液位和水流的动态方程。
```
% Nonlinear Water Tank Model
function [xdot, y] = NonlinearWaterTankModel(x, u, Ts)
% System Parameters
A1 = 0.5; A2 = 0.5; g = 9.81;
% State Equations
h1dot = (1/A1)*u - (1/A1)*sqrt(2*g)*sqrt(x(1));
h2dot = (1/A2)*sqrt(2*g)*sqrt(x(1)) - (1/A2)*sqrt(2*g)*sqrt(x(2));
% State Vector
xdot = [h1dot; h2dot];
% Output Equation
y = [x(1); x(2)];
end
```
2. 将非线性动态模型转换为离散时间的状态空间模型,其中状态向量包括液位和水流。
```
% Discretized State-Space Model
sys = c2d(@(x,u) NonlinearWaterTankModel(x,u,Ts),Ts);
A = sys.A; B = sys.B; C = sys.C; D = sys.D;
```
3. 使用NMPC算法进行控制设计,生成MATLAB函数脚本。
```
% Nonlinear MPC Controller
import casadi.*
% System Parameters
A1 = 0.5; A2 = 0.5; g = 9.81;
% Control Horizon
N = 10; Ts = 0.1;
% Prediction Horizon
T = N*Ts;
% State and Control Variables
x = MX.sym('x',2);
u = MX.sym('u');
% Initial State
x0 = MX.sym('x0',2);
% Reference Trajectory
r = MX.sym('r',2);
% Objective Function
J = 0;
% State Constraints
g = [];
% Control Constraints
h = [];
% NMPC Loop
for k = 1:N
% Construct the State Prediction
xk = x;
for j = 1:k
xk = xk + Ts*(A*xk + B*u);
end
% Construct the Objective Function
ek = xk - r;
J = J + ek.'*ek;
% Construct the State Constraints
g = [g; xk(1) >= 0; xk(2) >= 0];
% Construct the Control Constraints
h = [h; u >= 0.1; u <= 1];
end
% Construct the NMPC Controller
nlp = struct('x',vertcat(x,u),'f',J,'g',vertcat(g,h));
solver = nlpsol('solver','ipopt',nlp);
% NMPC Function
function [u, cost] = NonlinearMPC(x0,r)
% Construct the NMPC Constraints
args = struct;
args.lbg = 0;
args.ubg = 0;
args.lbx = [-inf;-inf;0.1];
args.ubx = [inf;inf;1];
args.x0 = [x0;0.1];
args.p = r;
% Solve the NMPC Problem
res = solver(args);
% Extract the Control Input
u = full(res.x(end));
% Extract the Cost Function
cost = full(res.f);
end
```
4. 在Simulink中导入NMPC控制器脚本,将其与非线性水箱系统模型进行集成。
<img src="https://img-blog.csdn.net/20160430144044456" width="600">
其中,NMPC控制器的代码可以在MATLAB Function模块中实现。
5. 进行仿真实验,验证NMPC控制器的性能和鲁棒性。
以下是完整的Simulink模型和NMPC控制器的代码:
Simulink模型:
<img src="https://img-blog.csdn.net/20160430144106894" width="600">
NMPC控制器代码:
```
% Nonlinear MPC Controller
import casadi.*
% System Parameters
A1 = 0.5; A2 = 0.5; g = 9.81;
% Control Horizon
N = 10; Ts = 0.1;
% Prediction Horizon
T = N*Ts;
% State and Control Variables
x = MX.sym('x',2);
u = MX.sym('u');
% Initial State
x0 = MX.sym('x0',2);
% Reference Trajectory
r = MX.sym('r',2);
% Objective Function
J = 0;
% State Constraints
g = [];
% Control Constraints
h = [];
% NMPC Loop
for k = 1:N
% Construct the State Prediction
xk = x;
for j = 1:k
xk = xk + Ts*(A*xk + B*u);
end
% Construct the Objective Function
ek = xk - r;
J = J + ek.'*ek;
% Construct the State Constraints
g = [g; xk(1) >= 0; xk(2) >= 0];
% Construct the Control Constraints
h = [h; u >= 0.1; u <= 1];
end
% Construct the NMPC Controller
nlp = struct('x',vertcat(x,u),'f',J,'g',vertcat(g,h));
solver = nlpsol('solver','ipopt',nlp);
% NMPC Function
function [u, cost] = NonlinearMPC(x0,r)
% Construct the NMPC Constraints
args = struct;
args.lbg = 0;
args.ubg = 0;
args.lbx = [-inf;-inf;0.1];
args.ubx = [inf;inf;1];
args.x0 = [x0;0.1];
args.p = r;
% Solve the NMPC Problem
res = solver(args);
% Extract the Control Input
u = full(res.x(end));
% Extract the Cost Function
cost = full(res.f);
end
```
阅读全文
相关推荐

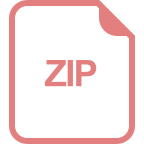
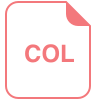
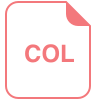
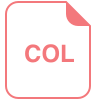
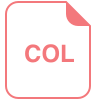
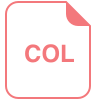
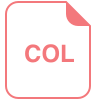
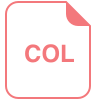
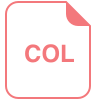
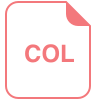
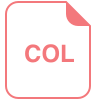
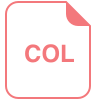
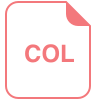
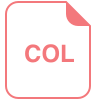