unity使用 public float radius = 1f; // 扇形的半径 public float angle = 90f; // 扇形的角度(以度为单位) public int segments = 24; // 扇形的分段数 private MeshFilter meshFilter; private MeshRenderer meshRenderer; 生成的扇形面由顶点向弧线发射射线,射线检测到的扇形面显示绿色,检测不到的显示红色,具体方法加注释
时间: 2024-03-27 08:36:03 浏览: 18
好的,这里提供一个利用射线检测方法生成扇形面的示例代码,并加上注释说明:
```csharp
using UnityEngine;
[RequireComponent(typeof(MeshFilter), typeof(MeshRenderer))]
public class FanShape : MonoBehaviour
{
public float radius = 1f; // 扇形的半径
public float angle = 90f; // 扇形的角度(以度为单位)
public int segments = 24; // 扇形的分段数
private MeshFilter meshFilter;
private MeshRenderer meshRenderer;
private Mesh mesh;
void Start()
{
meshFilter = GetComponent<MeshFilter>();
meshRenderer = GetComponent<MeshRenderer>();
mesh = new Mesh();
meshFilter.mesh = mesh;
// 生成扇形面的顶点数组
Vector3[] vertices = new Vector3[segments + 1];
vertices[0] = Vector3.zero;
float anglePerSegment = angle / segments;
for (int i = 1; i <= segments; i++)
{
float angleRad = Mathf.Deg2Rad * (i * anglePerSegment);
vertices[i] = new Vector3(Mathf.Cos(angleRad), 0f, Mathf.Sin(angleRad)) * radius;
}
// 生成扇形面的三角形索引数组
int[] triangles = new int[segments * 3];
for (int i = 0; i < segments; i++)
{
triangles[i * 3] = 0;
triangles[i * 3 + 1] = i + 1;
triangles[i * 3 + 2] = i == segments - 1 ? 1 : i + 2;
}
mesh.vertices = vertices;
mesh.triangles = triangles;
// 生成初始颜色数组
Color[] colors = new Color[vertices.Length];
for (int i = 0; i < colors.Length; i++)
{
colors[i] = Color.red;
}
mesh.colors = colors;
}
void Update()
{
// 射线检测并更新颜色
RaycastHit hit;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
if (Physics.Raycast(ray, out hit))
{
Vector3 hitPoint = transform.InverseTransformPoint(hit.point); // 将世界坐标系坐标转换为本地坐标系坐标
Vector2 hitPoint2D = new Vector2(hitPoint.x, hitPoint.z); // 将坐标系限制在 x-z 平面上
float hitAngle = Mathf.Atan2(hitPoint2D.y, hitPoint2D.x) * Mathf.Rad2Deg; // 计算射线击中点的角度
while (hitAngle < 0f) hitAngle += 360f;
hitAngle %= 360f;
float deltaAngle = angle / segments;
for (int i = 0; i <= segments; i++)
{
float angleRad = Mathf.Deg2Rad * (i * deltaAngle);
Vector2 vertex2D = new Vector2(Mathf.Cos(angleRad), Mathf.Sin(angleRad)) * radius;
if (Vector2.Dot(vertex2D, hitPoint2D) >= radius * Mathf.Cos(deltaAngle * Mathf.Deg2Rad))
{
mesh.colors[i] = Color.green; // 将射线检测到的扇形面的颜色设置为绿色
}
else
{
mesh.colors[i] = Color.red; // 将射线未检测到的扇形面的颜色设置为红色
}
}
mesh.colors[0] = Color.yellow; // 将扇形面中心的颜色设置为黄色
}
else
{
// 当射线没有击中物体时,将所有扇形面的颜色重置为红色
Color[] colors = new Color[mesh.colors.Length];
for (int i = 0; i < colors.Length; i++)
{
colors[i] = Color.red;
}
mesh.colors = colors;
}
mesh.RecalculateNormals(); // 重新计算法线
}
}
```
以上代码中,首先在 Start() 方法中生成了扇形面的顶点数组和三角形索引数组,并通过 mesh.vertices 和 mesh.triangles 将其赋值给 Mesh 对象。同时,还生成了初始颜色数组,将其通过 mesh.colors 设置给 Mesh 对象。
在 Update() 方法中,通过 Physics.Raycast 方法检测鼠标射线是否与扇形面相交,并计算出射线击中点的角度。然后循环遍历扇形面的顶点,对于每个顶点,计算出其对应的角度,通过 Vector2.Dot 方法判断该点是否在射线照射范围内,将射线检测到的扇形面的颜色设置为绿色,未检测到的设置为红色。最后,将扇形面中心的颜色设置为黄色。如果射线没有击中物体,则将所有扇形面的颜色重置为红色。最后,通过 mesh.RecalculateNormals() 重新计算法线,以确保扇形面的光照效果正确。
相关推荐
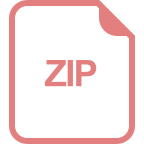
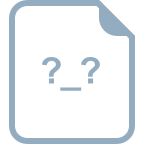
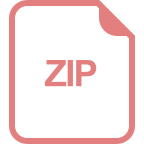
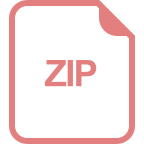
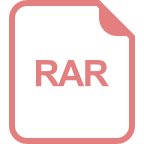
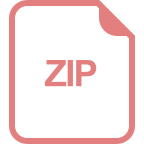
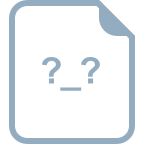
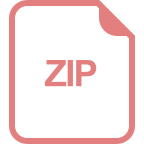
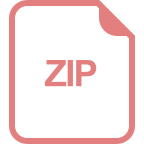
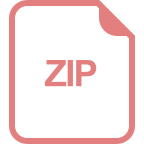
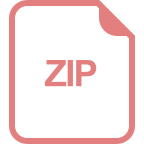