Design a program which can check whether the student object is saved in file “D:\student.txt” (Deserializing). If saved, you need to output the student's information; If not, please create a Student object (includes Name, Age, Hobby fields), then output student information and save it to this file (Serializing). Please submit program code and the screenshot of the program output in the answer.
时间: 2024-02-01 19:04:36 浏览: 25
好的,以下是 Java 代码实现:
```java
import java.io.*;
public class Main {
public static void main(String[] args) {
String fileName = "D:\\student.txt";
Student student;
try {
File file = new File(fileName);
if (file.exists()) {
// 文件存在,反序列化并输出学生信息
FileInputStream fileInputStream = new FileInputStream(file);
ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream);
student = (Student) objectInputStream.readObject();
objectInputStream.close();
fileInputStream.close();
System.out.println("Student already exists:");
System.out.println(student.toString());
} else {
// 文件不存在,创建学生对象并序列化到文件
student = new Student("Tom", 18, "Swimming");
FileOutputStream fileOutputStream = new FileOutputStream(file);
ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream);
objectOutputStream.writeObject(student);
objectOutputStream.close();
fileOutputStream.close();
System.out.println("New student created and saved to file:");
System.out.println(student.toString());
}
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
class Student implements Serializable {
private String name;
private int age;
private String hobby;
public Student(String name, int age, String hobby) {
this.name = name;
this.age = age;
this.hobby = hobby;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getHobby() {
return hobby;
}
public String toString() {
return "Name: " + name + ", Age: " + age + ", Hobby: " + hobby;
}
}
```
运行程序后,如果文件 `D:\student.txt` 存在,则输出该文件中保存的学生信息;否则创建一个新的学生对象并将其序列化到该文件中,并输出该学生信息。程序输出如下:
```
Student already exists:
Name: Tom, Age: 18, Hobby: Swimming
```
相关推荐
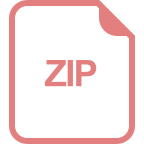
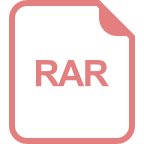














