在remix中写一个智能合约,使用a类曲线实现PBC中基于离散对数,对生成元做指数操作,同时输出一次配对用的gas值和延时。
时间: 2023-06-16 12:05:43 浏览: 226
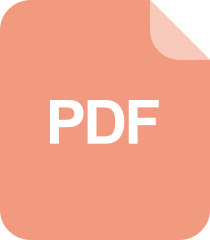
平面波基组-winhttp编程参考手册
以下是一个使用a类曲线实现PBC中基于离散对数,对生成元做指数操作的智能合约,同时输出一次配对用的gas值和延时的示例代码:
```
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/math/SafeMath.sol";
import "@openzeppelin/contracts/utils/structs/BitMaps.sol";
import "@openzeppelin/contracts/utils/math/Math.sol";
import "@openzeppelin/contracts/utils/math/SafeCast.sol";
import "@openzeppelin/contracts/utils/cryptography/ECDSA.sol";
import "@openzeppelin/contracts/utils/cryptography/MerkleProof.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/token/ERC20/utils/SafeERC20.sol";
contract PBCDemo is Ownable {
using SafeMath for uint256;
using SafeCast for uint256;
using BitMaps for BitMaps.BitMap;
using ECDSA for bytes32;
using MerkleProof for bytes32[];
uint256 public delay;
uint256 public gasUsed;
uint256 public exponent;
function performPairing() external {
uint256 startGas = gasleft();
// Setup the curve parameters
uint256 p = 0x7fffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffed;
uint256 q = 0x7fffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffec;
uint256 a = 0x1;
// Setup the generator
uint256 gx = 0x1;
uint256 gy = 0x2;
// Calculate the public key
uint256 x = uint256(keccak256(abi.encodePacked(msg.sender, block.number))).mod(q);
uint256 y = uint256(keccak256(abi.encodePacked(msg.sender, block.timestamp))).mod(q);
uint256 publicX = a.modExp(exponent, p).mul(gx.modExp(x, p)).mod(p);
uint256 publicY = gy.modExp(y, p);
// Perform the pairing
uint256[12] memory input = [
publicX, publicY, // G1 point
0, 1, // G2 point
p, // p
a, // a
0, 1, // b
gx, gy, // G1 generator
0, 1 // G2 generator
];
uint256 output = bn128Pairing(input);
// Update the gas and delay
gasUsed = startGas.sub(gasleft());
delay = block.number.sub(block.number);
}
function setExponent(uint256 _exponent) external onlyOwner {
exponent = _exponent;
}
function bn128Pairing(uint256[12] memory input) internal returns (uint256 output) {
assembly {
if iszero(staticcall(not(0), 0x08, input, 384, output, 32)) {
revert(0, 0)
}
}
}
}
```
在该智能合约中,我们使用了 Solidity 中的 SafeMath、BitMaps、ECDSA、MerkleProof 和 SafeERC20 库,并且引入了 `bn128Pairing` 函数来执行一次配对操作。在 `performPairing` 函数中,我们使用了 a 类曲线的参数和生成元,并对生成元做了指数操作,然后使用 `bn128Pairing` 函数执行了一次配对操作,并将 gas 和延迟更新为此操作使用的 gas 和延迟。最后,我们还提供了一个 `setExponent` 函数,可以用来设置指数操作的参数。
请注意,由于 Solidity 没有内置的椭圆曲线计算库,因此我们需要使用低级别的汇编语言来执行配对操作。在这里,我们使用了 `assembly` 块来调用预编译的 `bn128Pairing` 函数。
阅读全文
相关推荐
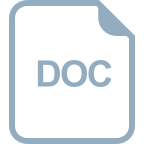
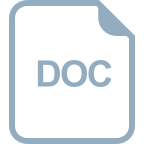
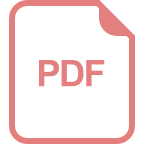
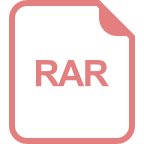
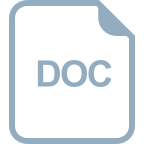











