如何将dataframe groupby转化为Series类型
时间: 2023-06-03 16:04:56 浏览: 216
可以使用groupby之后的agg函数来对分组后的数据进行计算,生成一个Series类型的结果。例如:
```
import pandas as pd
# 创建一个示例DataFrame
df = pd.DataFrame({
'key': ['A', 'B', 'C', 'A', 'B', 'C'],
'value': [1, 2, 3, 4, 5, 6]
})
# 使用groupby和agg进行分组计算
result = df.groupby('key')['value'].agg('sum')
# 打印结果
print(result)
```
输出结果为:
```
key
A 5
B 7
C 9
Name: value, dtype: int64
```
这个结果就是一个Series类型的对象,它的索引是分组的键值,值是计算后的结果。
相关问题
python如何对groupby()[]函数运行后的series转化为DataFrame型
在Python的Pandas库中,`groupby()`函数通常用于数据分组操作,它会返回一个GroupBy对象,该对象包含了分组后的Series。如果你想要将分组后的Series转换为DataFrame,可以按照以下步骤进行:
1. 使用`groupby()`对数据集进行分组。
```python
df.groupby('column_name') # 将'column_name'替换为你想分组的列名
```
2. 对每个分组应用聚合函数,如求和、平均等,并存储结果为Series。
```python
grouped = df.groupby('column_name').agg({'target_column': 'sum'}) # 'target_column'替换为你要汇总的列名
```
3. 将Series转换为DataFrame,可以使用`reset_index()`方法,这会添加一个新的索引级别并将Series变成DataFrame的行。
```python
result_df = grouped.reset_index()
```
现在,`result_df`就是分组后基于指定列的DataFrame形式。
python dataframe的group by
Python中的DataFrame可以使用groupby方法进行分组操作。通过groupby方法,可以将数据按照指定的列进行分组,然后对每个组进行聚合操作。
在使用groupby方法时,你可以传入一个列名或者一个Series对象来指定要分组的列。例如,使用df.groupby(df['key1'])可以按照'key1'列进行分组。这将返回一个GroupBy对象,可以对其进行进一步的聚合操作。
另外,你还可以同时传入多个列名或Series对象来进行多级分组。例如,使用df.groupby([df['key1'], df['key2']])可以按照'key1'和'key2'两列进行分组。这样得到的GroupBy对象可以对每个组进行聚合操作。
对于GroupBy对象,你可以调用各种聚合函数,如sum、mean、count等,以对每个组进行计算和统计。例如,使用gb.sum()可以对每个组进行求和操作。
此外,你还可以将groupby的结果转换为字典形式,通过dict(list(gb))可以将每个组的数据转化为字典,并以组名作为字典的键。
综上所述,通过groupby方法可以实现按照指定列进行分组,并进行各种聚合操作和数据转换。
阅读全文
相关推荐
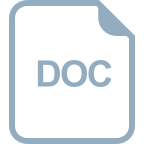
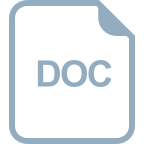
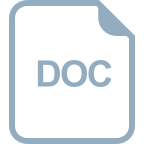
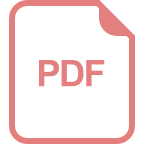
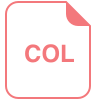
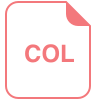
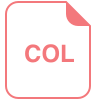
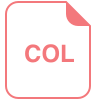
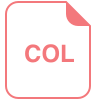
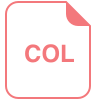
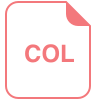





