class Complex{ double real, imag; public: Complex(double r=0, double i=0): real(r), imag(i){ } friend istream& operator>>(istream& in, Complex& c); //已实现 friend ostream& operator<<(ostream& out, const Complex& c);//已实现 friend Complex operator+(const Complex& c1, const Complex& c2); friend Complex operator-(const Complex& c1, const Complex& c2); Complex& operator+=(const Complex& c); Complex& operator-=(const Complex& c); };#include <iostream> using namespace std; int main() { Complex c1, c2; cin >> c1 >> c2; cout << c1 + c2 << endl; cout << c1 - c2 << endl; c1 += c2; cout << c1 << endl; c1 -= c2; cout << c1 << endl; return 0; } /* 请在这里填写答案,类的详细定义已由系统给出,并重载了输入输出运算符,你只需要写出需要重载的算术运算符函数即可 */
时间: 2023-06-17 10:05:44 浏览: 68
重载的算术运算符函数如下所示:
```c++
Complex operator+(const Complex& c1, const Complex& c2){
return Complex(c1.real+c2.real, c1.imag+c2.imag);
}
Complex operator-(const Complex& c1, const Complex& c2){
return Complex(c1.real-c2.real, c1.imag-c2.imag);
}
Complex& Complex::operator+=(const Complex& c){
real += c.real;
imag += c.imag;
return *this;
}
Complex& Complex::operator-=(const Complex& c){
real -= c.real;
imag -= c.imag;
return *this;
}
```
其中,`operator+` 重载了加法运算符,返回两个复数相加的结果;`operator-` 重载了减法运算符,返回两个复数相减的结果;`operator+=` 重载了复合赋值加法运算符,将一个复数加上另一个复数并返回结果;`operator-=` 重载了复合赋值减法运算符,将一个复数减去另一个复数并返回结果。
相关问题
#include<iostream>class Complex {private: double real; double imag;public: Complex(double r=0.0, double i=0.0) { real = r; imag = i; } Complex operator+(Complex c2); Complex operator-(Complex c2); void display();};Complex Complex::operator+(Complex c2) { Complex c; c.real = real + c2.real; c.imag = imag + c2.imag; return c;}Complex Complex::operator-(Complex c2) { Complex c; c.real = real - c2.real; c.imag = imag - c2.imag; return c;}void Complex::display() { std::cout << "(" << real << "," << imag << ")" << std::endl;}int main() { Complex c1(4, 3), c2(2, 1), c3(0, 0); std::cout << "c1="; c1.display(); std::cout << "c2="; c2.display(); c3 = c1 - c2; std::cout << "c3="; c3.display(); c3 = c1 + c2; std::cout << "c3="; c3.display(); return 0;} 要求重载运算符函数为友元函数
#include<iostream>class Complex {private: double real; double imag;public: Complex(double r=0.0, double i=0.0) { real = r; imag = i; } friend Complex operator+(Complex c1, Complex c2); friend Complex operator-(Complex c1, Complex c2); void display();};Complex operator+(Complex c1, Complex c2) { Complex c; c.real = c1.real + c2.real; c.imag = c1.imag + c2.imag; return c;}Complex operator-(Complex c1, Complex c2) { Complex c; c.real = c1.real - c2.real; c.imag = c1.imag - c2.imag; return c;}void Complex::display() { std::cout << "(" << real << "," << imag << ")" << std::endl;}int main() { Complex c1(4, 3), c2(2, 1), c3(0, 0); std::cout << "c1="; c1.display(); std::cout << "c2="; c2.display(); c3 = c1 - c2; std::cout << "c3="; c3.display(); c3 = c1 + c2; std::cout << "c3="; c3.display(); return 0;}
用C++给下面的代码写运行部分。class COMPLEX {private: double real; double imag;public: COMPLEX(double r = 0, double i = 0) : real(r), imag(i) {} COMPLEX operator+(const COMPLEX& rhs) const { return COMPLEX(real + rhs.real, imag + rhs.imag); } COMPLEX operator-(const COMPLEX& rhs) const { return COMPLEX(real - rhs.real, imag - rhs.imag); } COMPLEX& operator=(const COMPLEX& rhs) { real = rhs.real; imag = rhs.imag; return *this; }};
以下是用C++编写的运行部分:
```c++
#include <iostream>
using namespace std;
class COMPLEX {
private:
double real;
double imag;
public:
COMPLEX(double r = 0, double i = 0) : real(r), imag(i) {}
COMPLEX operator+(const COMPLEX& rhs) const {
return COMPLEX(real + rhs.real, imag + rhs.imag);
}
COMPLEX operator-(const COMPLEX& rhs) const {
return COMPLEX(real - rhs.real, imag - rhs.imag);
}
COMPLEX& operator=(const COMPLEX& rhs) {
real = rhs.real;
imag = rhs.imag;
return *this;
}
};
int main() {
COMPLEX a(1, 2), b(3, 4), c;
c = a + b;
cout << c.real << " + " << c.imag << "i" << endl;
c = a - b;
cout << c.real << " + " << c.imag << "i" << endl;
return 0;
}
```
在上述代码中,我们定义了一个名为`COMPLEX`的类,它表示一个复数,具有实部和虚部两个成员变量。我们使用构造函数初始化这两个成员变量,并且使用运算符重载函数`operator+`和`operator-`分别实现了复数的加法和减法。同时,我们也定义了一个赋值运算符`operator=`,用于将一个复数赋值给另一个复数。
在`main()`函数中,我们创建了两个复数对象`a`和`b`,并将它们相加和相减,将结果分别赋值给复数对象`c`。最后,我们输出了`c`的实部和虚部,以验证我们的代码是否正确。
相关推荐
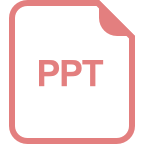
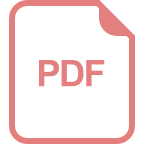
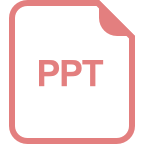











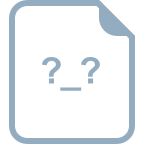
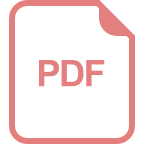