#include<iostream> using namespace std; class Complex { double real,imag; public: Complex(double r=0,double i=0):real(r),imag(i) {} //只是让代码看起来简短而已 double get_real() {return real;} double get_imag() {return imag;} void print_complex() {cout<<real<<"+"<<"j"<<imag<<endl;} Complex operator + (double a); Complex operator + (Complex x); friend ostream &operator << (ostream &out,Complex &A ); } ostream &operator << (ostream &out,Complex &A ) { out<<A.real<<"+"<<A.imag<<"j"; return out; } Complex Complex:: operator + (double a) { Complex m; m.real=real+a;//实数 m.imag=imag; return m; } Complex Complex::operator +(Complex x)//加不加都可以,引用调用 { Complex t; t.imag=imag+x.imag; t.real=real+x.real; return t; } int main() { Complex c1(1,2),c2(4,5),c3; c1=c1+4.2; //重载 复数类+整型 cout<<c1<<endl; cout<<c2<<endl; c3=c1+c2; //重载 复述类+复数类 cout<<c3<<endl; return 0; } 哪里有问题以及怎么解决
时间: 2023-12-10 09:42:13 浏览: 34
这段代码中有两个问题:
1. 在重载<<运算符时,应该将Complex对象作为常量引用传递,即:
```
friend ostream &operator << (ostream &out, const Complex &A );
```
2. 在Complex类的构造函数中,应该使用成员初始化列表来初始化real和imag成员变量,即:
```
Complex(double r=0, double i=0): real(r), imag(i) {}
```
修改后的代码如下:
```
#include<iostream>
using namespace std;
class Complex {
double real, imag;
public:
Complex(double r=0, double i=0): real(r), imag(i) {}
double get_real() {return real;}
double get_imag() {return imag;}
void print_complex() {cout<<real<<"+"<<"j"<<imag<<endl;}
Complex operator + (double a);
Complex operator + (Complex x);
friend ostream &operator << (ostream &out, const Complex &A );
};
ostream &operator << (ostream &out, const Complex &A ) {
out<<A.real<<"+"<<A.imag<<"j";
return out;
}
Complex Complex:: operator + (double a) {
Complex m;
m.real=real+a; //实数
m.imag=imag;
return m;
}
Complex Complex::operator +(Complex x) {
Complex t;
t.imag=imag+x.imag;
t.real=real+x.real;
return t;
}
int main() {
Complex c1(1,2),c2(4,5),c3;
c1=c1+4.2; //重载 复数类+整型
cout<<c1<<endl;
cout<<c2<<endl;
c3=c1+c2; //重载 复数类+复数类
cout<<c3<<endl;
return 0;
}
```
相关推荐
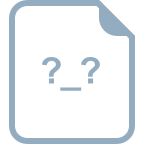















