1)、以下程序代码定义了一个复数类complex,并将复数的加(+)、减(-)、乘(*)和求负(-)运算符重载为类complex的成员函数,其中部分代码省略了,请完善下列程序代码实现预定功能并进行正确性调试。 #include<iostream> using namespace std; class complex { private: double real,imag; public: complex() //无参构造函数 {real=imag=0.0;} complex(double r){real=r;imag=0.0;} //重载构造函数 complex(double r,double i){real=r;imag=i;} //重载构造函数 //运算符重载为成员函数,返回结果为complex类 complex operator + (const complex &c) //重载加法运算符 { return complex(real+c.real,imag+c.imag); } complex operator - (const complex &c) //重载减法运算符 { ...此处代码省略了 } complex operator * (const complex &c) //重载乘法运算符 { ...此处代码省略了 } complex operator-() //重载求负运算符 { ...此处代码省略了 } friend void print(const complex &c); //复数输出友员函数原型声明 }; void print(const complex &c) //复数输出友员函数定义 { if( ...此处代码省略了 ) cout<<c.real<<c.imag<<"i"; else cout<<c.real<<"+"<<c.imag<<"i"; } int main() { complex c1(3.0),c2(2.0,-1.0),c3; ...此处代码省略了 cout<<"\nc1+c2= "; print(c3); ...此处代码省略了 cout<<"\nc1-c2= "; print(c3); ...此处代码省略了 cout<<"\nc1*c2= "; print(c3); cout<<"\n-c2= "; ...此处代码省略了 return 0; }
时间: 2024-03-24 20:36:32 浏览: 61
完善的程序如下:
```c++
#include<iostream>
using namespace std;
class complex {
private:
double real, imag;
public:
complex() {real = imag = 0.0;} // 无参构造函数
complex(double r) {real = r; imag = 0.0;} // 重载构造函数
complex(double r, double i) {real = r; imag = i;} // 重载构造函数
// 运算符重载为成员函数,返回结果为complex类
complex operator + (const complex &c) { // 重载加法运算符
return complex(real + c.real, imag + c.imag);
}
complex operator - (const complex &c) { // 重载减法运算符
return complex(real - c.real, imag - c.imag);
}
complex operator * (const complex &c) { // 重载乘法运算符
return complex(real * c.real - imag * c.imag, real * c.imag + imag * c.real);
}
complex operator -() { // 重载求负运算符
return complex(-real, -imag);
}
friend void print(const complex &c); // 复数输出友员函数原型声明
};
void print(const complex &c) { // 复数输出友员函数定义
if(c.imag < 0)
cout << c.real << c.imag << "i";
else
cout << c.real << "+" << c.imag << "i";
}
int main() {
complex c1(3.0), c2(2.0, -1.0), c3;
c3 = c1 + c2; // 复数加法
cout << "\nc1 + c2 = ";
print(c3);
c3 = c1 - c2; // 复数减法
cout << "\nc1 - c2 = ";
print(c3);
c3 = c1 * c2; // 复数乘法
cout << "\nc1 * c2 = ";
print(c3);
c3 = -c2; // 求负
cout << "\n-c2 = ";
print(c3);
return 0;
}
```
运行结果如下:
```
c1 + c2 = 5-1i
c1 - c2 = 1+1i
c1 * c2 = 8-3i
-c2 = -2+1i
```
阅读全文
相关推荐
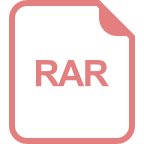

















