利用c++写一个程序,完整定义复数类Complex,在其中重载常见运算符,如“+”“-”“++”“--”“+=”,并且重载“==”和“!=”,使之能够比较两个复数
时间: 2023-11-09 22:07:02 浏览: 52
下面是一个示例代码,实现了复数类Complex的定义和常见运算符的重载。
```c++
#include <iostream>
class Complex {
private:
double real, imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& c) const {
return Complex(real + c.real, imag + c.imag);
}
Complex operator-(const Complex& c) const {
return Complex(real - c.real, imag - c.imag);
}
Complex operator++() {
real++;
return *this;
}
Complex operator++(int) {
Complex c(*this);
++(*this);
return c;
}
Complex operator--() {
real--;
return *this;
}
Complex operator--(int) {
Complex c(*this);
--(*this);
return c;
}
Complex& operator+=(const Complex& c) {
real += c.real;
imag += c.imag;
return *this;
}
bool operator==(const Complex& c) const {
return real == c.real && imag == c.imag;
}
bool operator!=(const Complex& c) const {
return !(*this == c);
}
friend std::ostream& operator<<(std::ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
};
int main() {
Complex c1(1, 2), c2(3, 4);
std::cout << "c1 = " << c1 << std::endl;
std::cout << "c2 = " << c2 << std::endl;
Complex c3 = c1 + c2;
std::cout << "c3 = " << c3 << std::endl;
Complex c4 = c1 - c2;
std::cout << "c4 = " << c4 << std::endl;
c1++;
std::cout << "c1++ = " << c1 << std::endl;
++c1;
std::cout << "++c1 = " << c1 << std::endl;
c1--;
std::cout << "c1-- = " << c1 << std::endl;
--c1;
std::cout << "--c1 = " << c1 << std::endl;
c1 += c2;
std::cout << "c1 += c2 = " << c1 << std::endl;
std::cout << "c1 == c2 ? " << (c1 == c2) << std::endl;
std::cout << "c1 != c2 ? " << (c1 != c2) << std::endl;
return 0;
}
```
该程序定义了Complex类,其中包含了实部和虚部两个成员变量,以及一些常见运算符的重载实现。
在main函数中,我们创建了两个复数对象c1和c2,并且对它们进行了加减等运算,同时也测试了自增、自减、复合赋值等运算符的重载实现。最后,我们使用重载的“==”和“!=”运算符比较了两个复数对象的大小关系。
上述代码只是一个示例,实际上还有很多其他的运算符可以进行重载,比如乘法、除法等等。在使用时,我们可以根据具体需求进行选择和实现。
相关推荐
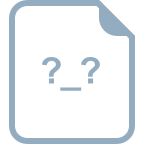














