用c++实现编写一个复数类的例子,重载 +、 - 、= 运算符,进而实现复数加、减、赋值运算
时间: 2023-10-20 10:12:19 浏览: 77
下面是一个使用C++实现复数类的例子,其中重载了+、-、=运算符,实现了复数的加、减、赋值运算:
```c++
#include <iostream>
using namespace std;
class Complex {
public:
Complex() : re(0), im(0) {} // 默认构造函数
Complex(double r, double i) : re(r), im(i) {} // 带参数的构造函数
// 重载加号运算符
Complex operator+(const Complex& c) const {
return Complex(re + c.re, im + c.im);
}
// 重载减号运算符
Complex operator-(const Complex& c) const {
return Complex(re - c.re, im - c.im);
}
// 重载赋值运算符
Complex& operator=(const Complex& c) {
re = c.re;
im = c.im;
return *this;
}
// 打印复数
void print() const {
cout << "(" << re << ", " << im << ")" << endl;
}
private:
double re, im; // 复数的实部和虚部
};
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
Complex c3 = c1 + c2; // 复数加法
c3.print(); // 输出 (4, 6)
Complex c4 = c1 - c2; // 复数减法
c4.print(); // 输出 (-2, -2)
c1 = c2; // 复数赋值
c1.print(); // 输出 (3, 4)
return 0;
}
```
在上面的代码中,我们定义了一个复数类`Complex`,并重载了+、-、=运算符,实现了复数的加、减、赋值运算。我们可以通过创建`Complex`类的对象来进行复数运算。
相关推荐
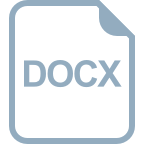
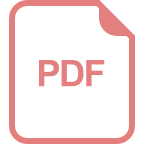
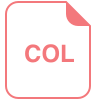













