使用C++编写一个复数类的例子,重载 +、 - 、= 运算符,进而实现复数加、减、赋值运算;
时间: 2024-01-26 18:04:56 浏览: 70
以下是一个简单的复数类的实现,包括重载 +、-、= 运算符,可以实现复数的加、减、赋值运算:
```cpp
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& c) const;
Complex operator-(const Complex& c) const;
Complex& operator=(const Complex& c);
friend ostream& operator<<(ostream& os, const Complex& c);
private:
double real;
double imag;
};
Complex Complex::operator+(const Complex& c) const
{
return Complex(this->real + c.real, this->imag + c.imag);
}
Complex Complex::operator-(const Complex& c) const
{
return Complex(this->real - c.real, this->imag - c.imag);
}
Complex& Complex::operator=(const Complex& c)
{
this->real = c.real;
this->imag = c.imag;
return *this;
}
ostream& operator<<(ostream& os, const Complex& c)
{
os << c.real << " + " << c.imag << "i";
return os;
}
int main()
{
Complex c1(1, 2);
Complex c2(3, 4);
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
Complex c3 = c1 + c2;
cout << "c3 = " << c3 << endl;
Complex c4 = c1 - c2;
cout << "c4 = " << c4 << endl;
c1 = c2;
cout << "c1 = " << c1 << endl;
return 0;
}
```
输出结果为:
```
c1 = 1 + 2i
c2 = 3 + 4i
c3 = 4 + 6i
c4 = -2 - 2i
c1 = 3 + 4i
```
可以看到,我们成功地实现了复数类,并且可以进行复数的加、减、赋值运算。
阅读全文
相关推荐
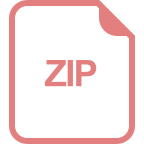
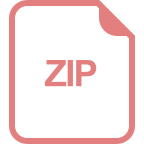
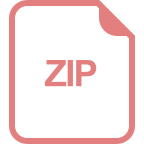
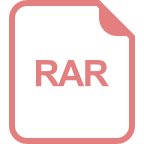
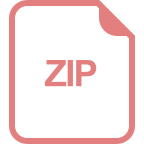
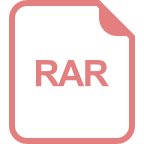
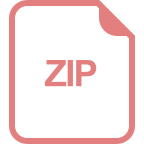
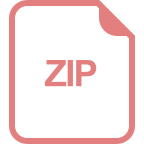
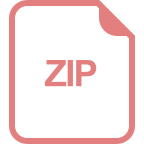
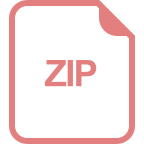
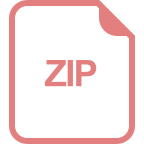