编写一个复数类的例子,重载 +、 - 、= 运算符,进而实现复数加、减、赋值运算;
时间: 2023-08-31 08:34:12 浏览: 87
### 回答1:
下面是一个简单的复数类的实现,其中重载了加、减、赋值运算符:
```c++
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
Complex operator+(const Complex& c) const {
return Complex(real + c.real, imag + c.imag);
}
Complex operator-(const Complex& c) const {
return Complex(real - c.real, imag - c.imag);
}
Complex& operator=(const Complex& c) {
if (this == &c)
return *this;
real = c.real;
imag = c.imag;
return *this;
}
void print() const {
cout << real << "+" << imag << "i" << endl;
}
private:
double real;
double imag;
};
int main() {
Complex a(1, 2);
Complex b(3, 4);
Complex c = a + b;
c.print();
Complex d = a - b;
d.print();
a = b;
a.print();
return 0;
}
```
在上述代码中,我们定义了一个 `Complex` 类,其中有两个私有成员变量 `real` 和 `imag` 分别表示复数的实部和虚部。我们通过重载加、减、赋值运算符,实现了复数的加、减、赋值运算。在 `main()` 函数中,我们创建了两个复数对象 `a` 和 `b`,然后通过重载的运算符实现了复数的加、减、赋值运算,并输出结果。
### 回答2:
复数是由实部和虚部组成的数,可以表示为a+bi的形式,其中a是实部,b是虚部,i是虚数单位,i^2为-1。
下面是一个复数类的例子,实现了重载+、-、=运算符,以及复数加减、赋值运算的功能:
```cpp
#include <iostream>
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex& operator=(const Complex& other) {
if (this == &other) {
return *this;
}
real = other.real;
imag = other.imag;
return *this;
}
void display() const {
std::cout << real << " + " << imag << "i" << std::endl;
}
};
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
Complex c3 = c1 + c2;
c3.display(); // 输出:4 + 6i
Complex c4 = c1 - c2;
c4.display(); // 输出:-2 - 2i
c1 = c2;
c1.display(); // 输出:3 + 4i
return 0;
}
```
这个例子中,我们定义了一个`Complex`类,其中包含了实部`real`和虚部`imag`两个私有成员变量。我们通过构造函数初始化这两个成员变量,构造函数的默认参数为0。
然后,我们重载了`+`、`-`、`=`运算符,通过成员函数的方式进行重载。`+`运算符用于实现两个复数相加,`-`运算符用于实现两个复数相减,`=`运算符用于实现赋值操作。它们返回一个新的`Complex`对象。
在`main`函数中,我们创建了两个复数对象`c1`和`c2`,然后通过对应的运算符对它们进行相加、相减和赋值操作,并通过`display`函数输出结果。
输出结果如注释所示。
### 回答3:
复数是由实部和虚部组成的数,可以用a+bi的形式表示,其中a为实部,b为虚部,i为虚数单位。为了实现复数的加、减以及赋值运算,可以定义一个复数类,重载+、-、=运算符。
下面是一个复数类的例子:
```
#include<iostream>
using namespace std;
class Complex {
private:
double real; // 实部
double imaginary; // 虚部
public:
Complex(double r = 0, double i = 0) {
real = r;
imaginary = i;
}
// 重载+运算符,实现复数的加法
Complex operator+(const Complex& c) const {
Complex res;
res.real = real + c.real;
res.imaginary = imaginary + c.imaginary;
return res;
}
// 重载-运算符,实现复数的减法
Complex operator-(const Complex& c) const {
Complex res;
res.real = real - c.real;
res.imaginary = imaginary - c.imaginary;
return res;
}
// 重载=运算符,实现复数的赋值
Complex& operator=(const Complex& c) {
if (this == &c) {
return *this;
}
real = c.real;
imaginary = c.imaginary;
return *this;
}
// 打印复数
void print() {
cout << real;
if (imagina
阅读全文
相关推荐










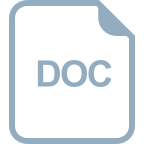
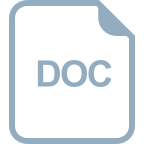
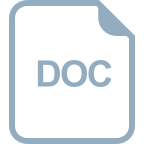


