#include <iostream> using namespace std; int main() { Complex c1, c2; cin >> c1 >> c2; cout << c1 + c2 << endl; cout << c1 - c2 << endl; c1 += c2; cout << c1 << endl; c1 -= c2; cout << c1 << endl; return 0; } /* 请在这里填写答案,类的详细定义已由系统给出,并重载了输入输出运算符,你只需要写出需要重载的算术运算符函数即可 */
时间: 2023-06-19 14:04:31 浏览: 81
根据代码中的提示,需要重载类 `Complex` 的算术运算符。假设 `Complex` 类的定义如下:
```cpp
class Complex {
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex& operator+=(const Complex& other) {
real += other.real;
imag += other.imag;
return *this;
}
Complex& operator-=(const Complex& other) {
real -= other.real;
imag -= other.imag;
return *this;
}
friend istream& operator>>(istream& is, Complex& c) {
is >> c.real >> c.imag;
return is;
}
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
private:
double real, imag;
};
```
则需要重载的算术运算符函数如下:
```cpp
Complex operator*(const Complex& c1, const Complex& c2) {
double real = c1.real * c2.real - c1.imag * c2.imag;
double imag = c1.real * c2.imag + c1.imag * c2.real;
return Complex(real, imag);
}
Complex operator/(const Complex& c1, const Complex& c2) {
double denominator = c2.real * c2.real + c2.imag * c2.imag;
double real = (c1.real * c2.real + c1.imag * c2.imag) / denominator;
double imag = (c1.imag * c2.real - c1.real * c2.imag) / denominator;
return Complex(real, imag);
}
```
其中,`operator*` 重载了乘法运算符,`operator/` 重载了除法运算符。它们都返回一个新的 `Complex` 对象,表示两个 `Complex` 对象的乘积或商。需要注意的是,除法运算符除数不能为零,此处没有进行特判。在实际使用中,需要根据实际情况进行处理。
阅读全文
相关推荐
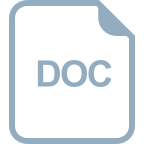
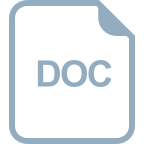
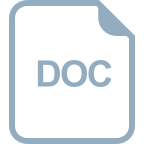















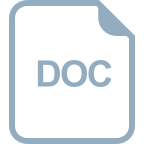