请完成Complex类的定义,使得下面的主函数能正常运行。 int main( ) { Complex c1(1,2); cout<<"c1="<<c1; //operator<<(cout,c1); Complex c2(2); // default argument is i=0 cout<<"c2="<<c2<<endl; Complex c3(c1); //invoke the copy constructor cout<<"c3="<<c3; c1=c1+c2+c3; // c1=(c1.operator+(c2)).operator+(c3); cout<<"c1=c1+c2+c3="<<c1; c2=-c3; // c2=c3.operator-(); cout<<"c2=-c3="<<c2; c3=c2-c1; // c3=c2.operator-(c1); cout<<"c3=c2-c1="<< c3; Complex r2=2+c2; // calls operator+ (2, c2) cout<<"r2=2+c2="<<r2; Complex c4; cin>>c4; cout<<"c4="<<c4; cout<<"the module of c4 is: "<<c4.GetMagnitude()<<endl;//计算复数的模 return 0; }
时间: 2023-10-30 09:11:43 浏览: 46
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 拷贝构造函数
Complex(const Complex& c) {
real = c.real;
imag = c.imag;
}
// 重载运算符 +
Complex operator+(const Complex& c) const {
return Complex(real + c.real, imag + c.imag);
}
// 重载运算符 -
Complex operator-(const Complex& c) const {
return Complex(real - c.real, imag - c.imag);
}
// 重载运算符 - (负号)
Complex operator-() const {
return Complex(-real, -imag);
}
// 重载运算符 + (用于实数与复数相加)
friend Complex operator+(double r, const Complex& c) {
return Complex(r + c.real, c.imag);
}
// 重载运算符 << (输出运算符)
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
// 重载运算符 >> (输入运算符)
friend istream& operator>>(istream& is, Complex& c) {
cout << "请输入实部和虚部(以空格分隔):";
is >> c.real >> c.imag;
return is;
}
// 计算复数的模
double GetMagnitude() const {
return sqrt(real * real + imag * imag);
}
};
int main() {
Complex c1(1, 2);
cout << "c1=" << c1 << endl;
Complex c2(2);
cout << "c2=" << c2 << endl;
Complex c3(c1);
cout << "c3=" << c3 << endl;
c1 = c1 + c2 + c3;
cout << "c1=c1+c2+c3=" << c1 << endl;
c2 = -c3;
cout << "c2=-c3=" << c2 << endl;
c3 = c2 - c1;
cout << "c3=c2-c1=" << c3 << endl;
Complex r2 = 2 + c2;
cout << "r2=2+c2=" << r2 << endl;
Complex c4;
cin >> c4;
cout << "c4=" << c4;
cout << "the module of c4 is: " << c4.GetMagnitude() << endl;
return 0;
}
```
相关推荐
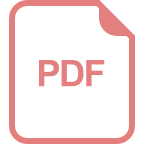
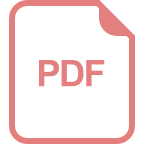
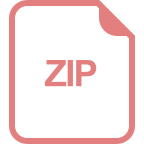














