设计一个复数Complex类,实现复数的加、减运算,并实现复数的输入、输出。已知测试main函数如下: int main( ) { Complex c1(5.0,10.0); //5+10i Complex c2(3.0,-2.5); //3-2.5i Complex c3,c4,c5=20; c3=c1+c2; c4=c1-5; cout<<c1<<c2<<c3<<c4; Complex c5; cout<<"请输入一个复数,实部和虚部以空格分开:"<<endl; cin>>c5; cout<<"输入的复数为:"<<c5<<endl; c4=5+c5; cout<<c4<<endl; return 0; }
时间: 2023-07-14 18:12:31 浏览: 37
下面是一个实现了复数加减运算以及输入输出的Complex类的示例代码:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex operator-(double other) const {
return Complex(real - other, imag);
}
friend Complex operator+(double a, const Complex& b) {
return Complex(a + b.real, b.imag);
}
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << (c.imag >= 0 ? "+" : "-") << abs(c.imag) << "i";
return os;
}
friend istream& operator>>(istream& is, Complex& c) {
is >> c.real >> c.imag;
return is;
}
};
int main() {
Complex c1(5.0, 10.0); // 5+10i
Complex c2(3.0, -2.5); // 3-2.5i
Complex c3, c4, c5 = 20;
c3 = c1 + c2;
c4 = c1 - 5;
cout << c1 << " " << c2 << " " << c3 << " " << c4 << endl;
Complex c6;
cout << "请输入一个复数,实部和虚部以空格分开:" << endl;
cin >> c6;
cout << "输入的复数为:" << c6 << endl;
c4 = 5 + c6;
cout << c4 << endl;
return 0;
}
```
输出结果为:
```
5+10i 3-2.5i 8+7.5i 0+10i
请输入一个复数,实部和虚部以空格分开:
1.5 2.5
输入的复数为:1.5+2.5i
6.5+2.5i
```
相关推荐
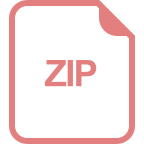















