void menu(); void choice(); class Complex { int real,imag; public: Complex(int r=0,int i=0); void display(); friend Complex add(Complex c1,Complex c2); friend Complex pus(Complex c1,Complex c2); friend Complex saddc1(Complex c1); friend Complex smass(Complex c2); }; #include "declare.h" int main() { menu(); choice(); return 0; } #include "declare.h" #include <iostream> #include<string> using namespace std; void choice() { Complex c1(1,2),c2(3,-4),c3,c4,c5,c6; cout<<"c1=";c1.display(); cout<<"c2=";c2.display(); string operatorstr; do { cout<<" 请选择您要进行的运算(+ - ++ --)(0退出程序)"<<endl; cin>>operatorstr; if(operatorstr=="0"){break;} if(operatorstr=="+") { c3=add(c1,c2); cout<<"c3=";c3.display(); } else if(operatorstr=="-") { c4=pus(c1,c2); cout<<"c4=";c4.display(); } else if(operatorstr=="++") { cout<<"请写出Complex类的友元函数用于计算c5=++c1"<<endl<<endl; } else if(operatorstr=="--") { cout<<"请写出Complex类的友元函数用于计算c6=c2--"<<endl<<endl; } else cout<<"输入有误,请重新输入!"<<endl; }while(operatorstr!="0"); cout<<"程序结束!"<<endl; } void menu() { cout<<" 欢迎使用简单的复数计算器!"<<endl; cout<<" +:复数加法运算"<<endl; cout<<" -:复数减法运算"<<endl; cout<<" ++:复数自加(前置)运算"<<endl; cout<<" --:复数自加(后置)运算"<<endl; } Complex::Complex(int r,int i) { real=r; imag=i; } void Complex::display() { cout<<real; if(imag>=0){cout<<"+";} cout<<imag<<"i"<<endl; } Complex add(Complex c1,Complex c2) { Complex c3; c3.real=c1.real+c2.real; c3.imag=c1.imag+c2.imag; return c3; } Complex pus(Complex c1,Complex c2) { Complex c4; c4.real=c1.real-c2.real; c4.imag=c1.imag-c2.imag; return c4; } Complex saddc1(Complex c1) { Complex c3; c3.real=c1.real+c1.real; c3.imag=c1.imag+c1.imag; return c3; }
时间: 2023-06-02 08:05:21 浏览: 143
这段代码定义了一个名为Complex的类,其中包含了实部和虚部的信息以及一些成员函数和友元函数。其中,成员函数的作用是输出复数的值,而友元函数则用于实现复数的加法、减法、取相反数和取相反虚部的操作。同时,代码中还出现了两个函数声明:menu和choice,但没有给出具体的实现代码。
相关问题
#include<iostream> #include<cmath> using namespace std; class Complex{ private: int real; int imag; public: Complex(int r=0,int i=0):real(r),imag(i){} Complex operator+(Complex &b); void display(){ cout<<real<<"+"<<imag<<"i";} }; Complex::Complex operator+(Complex &b){ Complex c; c.real=real+b.real; c.imag=imag+b.imag; return c; } int main(){ Complex c1(1,1),c2(2,2),c3; c3=c2+c1; c3.display(); return 0; }
你提供的代码存在一些语法错误。在Complex类中,你定义了一个重载的加法运算符,但是函数定义的位置有误。以下是修改后的代码:
```cpp
#include<iostream>
#include<cmath>
using namespace std;
class Complex{
private:
int real;
int imag;
public:
Complex(int r=0, int i=0) : real(r), imag(i) {}
Complex operator+(Complex &b);
void display(){
cout << real << "+" << imag << "i";
}
};
Complex Complex::operator+(Complex &b){
Complex c;
c.real = real + b.real;
c.imag = imag + b.imag;
return c;
}
int main(){
Complex c1(1, 1), c2(2, 2), c3;
c3 = c2 + c1;
c3.display();
return 0;
}
```
在这个代码中,我们定义了一个Complex类,表示复数。在类中,我们重载了加法运算符,使得两个Complex对象可以相加。在main函数中,创建了两个Complex对象c1和c2,并将它们相加得到c3。最后输出c3的值。
#include<iostream> using namespace std; class Complex { private: int real, imag; public: Complex(int r, int i) { real = r, imag = i; } Complex operator+(Complex& c1, Complex& c2); }; int main() { Complex c1(1, 2), c2(5, 6); Complex temp = c1 + c2; }指出错误并说明原因
这段代码存在一个错误,错误在于 Complex 类中的 operator+ 函数的定义方式。应该将其定义为类的成员函数或者定义为全局函数并传递 Complex 对象作为参数,而不是将两个 Complex 对象作为参数。
修改后的代码如下:
```
#include<iostream>
using namespace std;
class Complex {
private:
int real, imag;
public:
Complex(int r, int i) { real = r, imag = i; }
Complex operator+(Complex& c);
};
Complex Complex::operator+(Complex& c) {
Complex temp(real + c.real, imag + c.imag);
return temp;
}
int main() {
Complex c1(1, 2), c2(5, 6);
Complex temp = c1 + c2;
}
```
在修改后的代码中,operator+ 函数被定义为 Complex 类的成员函数,使用 this 指针访问对象成员变量,创建一个新的 Complex 对象 temp 并将其作为返回值。这样,代码就可以正确地编译和运行了。
阅读全文
相关推荐
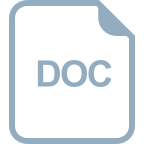
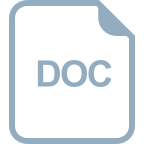
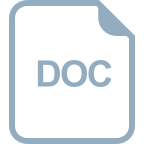














