用C++给下面的代码写运行部分。class COMPLEX {private: double real; double imag;public: COMPLEX(double r = 0, double i = 0) : real(r), imag(i) {} COMPLEX operator+(const COMPLEX& rhs) const { return COMPLEX(real + rhs.real, imag + rhs.imag); } COMPLEX operator-(const COMPLEX& rhs) const { return COMPLEX(real - rhs.real, imag - rhs.imag); } COMPLEX& operator=(const COMPLEX& rhs) { real = rhs.real; imag = rhs.imag; return *this; }};
时间: 2024-03-23 16:41:37 浏览: 157
以下是用C++编写的运行部分:
```c++
#include <iostream>
using namespace std;
class COMPLEX {
private:
double real;
double imag;
public:
COMPLEX(double r = 0, double i = 0) : real(r), imag(i) {}
COMPLEX operator+(const COMPLEX& rhs) const {
return COMPLEX(real + rhs.real, imag + rhs.imag);
}
COMPLEX operator-(const COMPLEX& rhs) const {
return COMPLEX(real - rhs.real, imag - rhs.imag);
}
COMPLEX& operator=(const COMPLEX& rhs) {
real = rhs.real;
imag = rhs.imag;
return *this;
}
};
int main() {
COMPLEX a(1, 2), b(3, 4), c;
c = a + b;
cout << c.real << " + " << c.imag << "i" << endl;
c = a - b;
cout << c.real << " + " << c.imag << "i" << endl;
return 0;
}
```
在上述代码中,我们定义了一个名为`COMPLEX`的类,它表示一个复数,具有实部和虚部两个成员变量。我们使用构造函数初始化这两个成员变量,并且使用运算符重载函数`operator+`和`operator-`分别实现了复数的加法和减法。同时,我们也定义了一个赋值运算符`operator=`,用于将一个复数赋值给另一个复数。
在`main()`函数中,我们创建了两个复数对象`a`和`b`,并将它们相加和相减,将结果分别赋值给复数对象`c`。最后,我们输出了`c`的实部和虚部,以验证我们的代码是否正确。
阅读全文
相关推荐
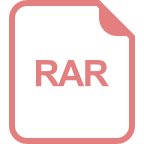
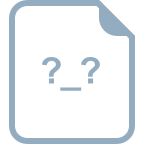
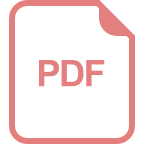
















