#include<iostream> using namespace std; //你提交的代码在这里 int main() { Complex c1; cout<<"Show object c1:"<<c1<<endl; double re,im; cin>>re>>im; Complex c2(re,im); cout<<"Show object c2:"<<c2<<endl; cin>>re>>im; c1.Set(re,im); cout<<"Reset and Show object c1:"<<c1<<endl; Complex c3; c3=c1+c2; cout<<c1<<" + "<<c2<<" = "<<c3<<endl; c3=c1-c2; cout<<c1<<" - "<<c2<<" = "<<c3<<endl; c3=c1*c2; cout<<c1<<" * "<<c2<<" = "<<c3<<endl; return 0; }
时间: 2023-08-20 08:39:49 浏览: 44
这段代码是一个关于复数类的程序,可以进行复数的加减乘运算,并且支持复数对象的输出。具体实现过程如下:
1. 定义了一个Complex类,包含了实部和虚部两个成员变量,以及一些成员函数,如构造函数、设置函数、加减乘运算符等。
2. 在主函数中,首先创建了一个默认的复数对象c1,并输出其值。
3. 接着通过用户输入的方式,创建了一个新的复数对象c2,并输出其值。
4. 再次通过用户输入的方式,重置了c1的值,并输出其值。
5. 然后定义了一个新的复数对象c3,通过运算符重载实现了c1与c2的加减乘运算,并输出运算结果。
6. 最后返回0,程序结束。
需要注意的是,复数的输出格式需要按照一定规则进行格式化,具体规则已在代码中给出。
相关问题
请设计复数类complex,实现复数的输入、输出和加法运算。\n\n#include <iostream>\n#include <iomanip>\nusing namespace std;\n\n/* 你提交的
这道题目要求我们设计一个名为complex的类来实现复数的输入、输出和加法运算。下面是代码实现:
#include <iostream>
#include <iomanip>
using namespace std;
class complex {
private:
double real;
double imag;
public:
complex(double a=0, double b=0) {
real = a;
imag = b;
}
friend istream& operator>>(istream& input, complex& c) {
input >> c.real >> c.imag;
return input;
}
friend ostream& operator<<(ostream& output, const complex& c) {
output << setiosflags(ios::fixed) << setprecision(2) << c.real << "+" << c.imag << "i";
return output;
}
friend complex operator+(const complex& c1, const complex& c2) {
complex temp;
temp.real = c1.real + c2.real;
temp.imag = c1.imag + c2.imag;
return temp;
}
};
int main() {
complex c1, c2;
cout << "请输入两个复数:";
cin >> c1 >> c2;
complex c3 = c1 + c2;
cout << "它们的和是:" << c3 << endl;
return 0;
}
解释一下代码:
我们定义了一个complex类来表示复数,其中包含了两个数据成员:实部(real)和虚部(imag)。在类中,我们重载了两个运算符:输入运算符>>和输出运算符<<。同时,我们还重载了加法运算符+来实现两个复数的加法运算。
在主函数中,我们先定义了两个complex类型的变量c1和c2,用于存储输入的两个复数。然后,我们利用重载的输入运算符>>来实现复数的输入。接着,我们定义了一个新的complex类型变量c3,将它赋值为c1与c2的和。最后,我们利用重载的输出运算符<<来输出c3的值,即两个复数的和。
这段代码实现了复数的输入、输出和加法运算,能够很好地满足题目要求。
#include <iostream> using namespace std; int main() { Complex c1, c2; cin >> c1 >> c2; cout << c1 + c2 << endl; cout << c1 - c2 << endl; c1 += c2; cout << c1 << endl; c1 -= c2; cout << c1 << endl; return 0; } /* 请在这里填写答案,类的详细定义已由系统给出,并重载了输入输出运算符,你只需要写出需要重载的算术运算符函数即可 */
根据代码中的提示,需要重载类 `Complex` 的算术运算符。假设 `Complex` 类的定义如下:
```cpp
class Complex {
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex& operator+=(const Complex& other) {
real += other.real;
imag += other.imag;
return *this;
}
Complex& operator-=(const Complex& other) {
real -= other.real;
imag -= other.imag;
return *this;
}
friend istream& operator>>(istream& is, Complex& c) {
is >> c.real >> c.imag;
return is;
}
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
private:
double real, imag;
};
```
则需要重载的算术运算符函数如下:
```cpp
Complex operator*(const Complex& c1, const Complex& c2) {
double real = c1.real * c2.real - c1.imag * c2.imag;
double imag = c1.real * c2.imag + c1.imag * c2.real;
return Complex(real, imag);
}
Complex operator/(const Complex& c1, const Complex& c2) {
double denominator = c2.real * c2.real + c2.imag * c2.imag;
double real = (c1.real * c2.real + c1.imag * c2.imag) / denominator;
double imag = (c1.imag * c2.real - c1.real * c2.imag) / denominator;
return Complex(real, imag);
}
```
其中,`operator*` 重载了乘法运算符,`operator/` 重载了除法运算符。它们都返回一个新的 `Complex` 对象,表示两个 `Complex` 对象的乘积或商。需要注意的是,除法运算符除数不能为零,此处没有进行特判。在实际使用中,需要根据实际情况进行处理。
相关推荐
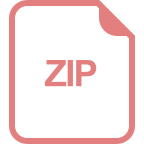
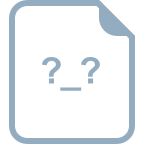













