在python中用opencv将一条折线图进行平滑处理
时间: 2023-05-11 18:01:47 浏览: 154
可以使用OpenCV中的函数cv2.GaussianBlur()对折线图进行平滑处理。具体步骤如下:
1. 读取折线图并转换为灰度图像。
2. 对灰度图像进行高斯模糊处理,使用cv2.GaussianBlur()函数,可以指定高斯核的大小和标准差。
3. 对处理后的图像进行二值化处理,可以使用cv2.threshold()函数。
4. 对二值化后的图像进行边缘检测,可以使用cv2.Canny()函数。
5. 对边缘检测后的图像进行霍夫直线变换,可以使用cv2.HoughLinesP()函数,得到折线的端点坐标。
6. 对折线进行平滑处理,可以使用numpy.polyfit()函数拟合一条曲线,然后使用numpy.polyval()函数计算平滑后的折线。
具体实现可以参考以下代码:
import cv2
import numpy as np
# 读取折线图并转换为灰度图像
img = cv2.imread('line.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 对灰度图像进行高斯模糊处理
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# 对处理后的图像进行二值化处理
ret, thresh = cv2.threshold(blur, 0, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)
# 对二值化后的图像进行边缘检测
edges = cv2.Canny(thresh, 50, 150, apertureSize=3)
# 对边缘检测后的图像进行霍夫直线变换
lines = cv2.HoughLinesP(edges, 1, np.pi/180, threshold=100, minLineLength=100, maxLineGap=10)
# 得到折线的端点坐标
points = []
for line in lines:
x1, y1, x2, y2 = line[0]
points.append((x1, y1))
points.append((x2, y2))
# 对折线进行平滑处理
x = [p[0] for p in points]
y = [p[1] for p in points]
coeffs = np.polyfit(x, y, 3)
smooth_y = np.polyval(coeffs, x)
# 绘制平滑后的折线
for i in range(len(x)-1):
cv2.line(img, (x[i], int(smooth_y[i])), (x[i+1], int(smooth_y[i+1])), (0, 0, 255), 2)
cv2.imshow('Smoothed Line', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
相关推荐
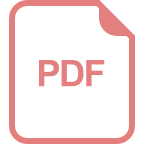
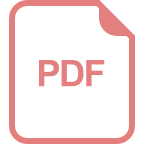
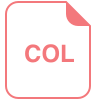
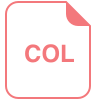
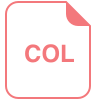
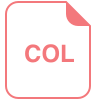
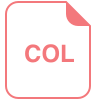









