Unity读取C:\Kistler\DynoWare\Data\26WCut_1.txt数据,以第一列为横坐标,第二列为纵坐标在Xcharts里面绘图
时间: 2023-06-28 11:13:14 浏览: 97
首先,需要将文本数据读取到Unity中。可以使用Unity的StreamReader类来实现:
```csharp
using System.IO;
// 读取文本文件
StreamReader reader = new StreamReader("C:/Kistler/DynoWare/Data/26WCut_1.txt");
// 存储横坐标和纵坐标
List<float> xValues = new List<float>();
List<float> yValues = new List<float>();
while (!reader.EndOfStream)
{
string line = reader.ReadLine();
string[] values = line.Split('\t');
xValues.Add(float.Parse(values[0]));
yValues.Add(float.Parse(values[1]));
}
reader.Close();
```
接下来,需要在Unity中使用XCharts插件来绘制图表。首先需要将XCharts的Prefab拖入场景中,并创建一个新的LineChart。
```csharp
using UnityEngine;
using UnityEngine.UI;
using XCharts;
public class DrawChart : MonoBehaviour
{
public GameObject chartPrefab;
public float chartWidth = 800f;
public float chartHeight = 600f;
private LineChart chart;
void Start()
{
// 创建图表
GameObject chartObject = Instantiate(chartPrefab);
chartObject.transform.SetParent(transform);
chartObject.GetComponent<RectTransform>().sizeDelta = new Vector2(chartWidth, chartHeight);
// 获取图表组件
chart = chartObject.GetComponent<LineChart>();
// 设置横坐标和纵坐标
chart.xAxis.data.Clear();
chart.yAxis.data.Clear();
chart.xAxis.data.AddRange(xValues);
chart.yAxis.data.AddRange(yValues);
// 添加数据
Series series = chart.series.Add(SeriesType.Line);
series.name = "Data";
for (int i = 0; i < xValues.Count; i++)
{
series.AddData(i, yValues[i]);
}
}
}
```
这样,就可以在Unity中绘制出对应的图表。需要注意的是,XCharts需要使用Unity的UI系统来渲染图表,所以需要将图表的Prefab添加到Canvas中。另外,也需要在Unity的Player Settings中设置Scripting Runtime Version为.NET 4.x Equivalent,否则会出现编译错误。
阅读全文
相关推荐


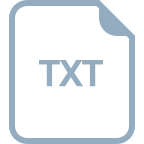








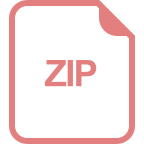
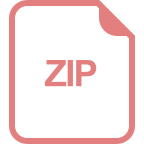