jt1078协议的G711a音频帧数据,用ffmpegRTMP推流,C++实现
时间: 2024-02-23 17:58:52 浏览: 21
要将jt1078协议的G711a音频帧数据通过ffmpeg RTMP推流,可以按照以下步骤实现:
1. 解析JT1078协议的音频帧数据,获取音频数据和音频帧头信息。
2. 使用FFmpeg库打开音频编码器,设置音频编码参数,创建音频编码器上下文。
3. 将JT1078协议的音频数据转换为PCM格式的音频数据。
4. 将PCM格式的音频数据送入音频编码器中,进行音频编码。
5. 创建FFmpeg的RTMP推流上下文,设置RTMP推流地址。
6. 将音频编码后的数据通过RTMP推流上下文,推送到RTMP服务器上。
以下是一个简单的C++代码示例:
```c++
#include <iostream>
#include <cstring>
#include <cstdlib>
#include <cstdio>
#include <stdint.h>
#include <unistd.h>
#include <signal.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <netinet/tcp.h>
#include <errno.h>
#include <time.h>
#include <math.h>
extern "C" {
#include "libavcodec/avcodec.h"
#include "libavformat/avformat.h"
#include "libswscale/swscale.h"
#include "libavutil/opt.h"
#include "libavutil/imgutils.h"
}
#define AUDIO_CODEC_ID AV_CODEC_ID_PCM_ALAW
#define AUDIO_SAMPLE_RATE 8000
#define AUDIO_BIT_RATE 64000
#define AUDIO_CHANNELS 1
#define RTMP_URL "rtmp://your_rtmp_url"
int main(int argc, char *argv[])
{
AVCodec *audio_codec = NULL;
AVCodecContext *audio_codec_ctx = NULL;
AVFrame *audio_frame = NULL;
AVPacket audio_packet;
AVFormatContext *rtmp_fmt_ctx = NULL;
AVOutputFormat *rtmp_fmt = NULL;
AVStream *audio_stream = NULL;
int ret = 0;
uint8_t *audio_data = NULL;
int audio_data_size = 0;
int audio_frame_size = 0;
int audio_frame_count = 0;
int64_t pts = 0;
int64_t last_audio_pts = 0;
// 1. 解析JT1078协议的音频帧数据,获取音频数据和音频帧头信息。
// ...
// 2. 使用FFmpeg库打开音频编码器,设置音频编码参数,创建音频编码器上下文。
audio_codec = avcodec_find_encoder(AUDIO_CODEC_ID);
if (!audio_codec) {
printf("Audio codec not found\n");
goto end;
}
audio_codec_ctx = avcodec_alloc_context3(audio_codec);
if (!audio_codec_ctx) {
printf("Could not allocate audio codec context\n");
goto end;
}
audio_codec_ctx->sample_fmt = audio_codec->sample_fmts[0];
audio_codec_ctx->bit_rate = AUDIO_BIT_RATE;
audio_codec_ctx->sample_rate = AUDIO_SAMPLE_RATE;
audio_codec_ctx->channels = AUDIO_CHANNELS;
ret = avcodec_open2(audio_codec_ctx, audio_codec, NULL);
if (ret < 0) {
printf("Could not open audio codec: %d\n", ret);
goto end;
}
audio_frame = av_frame_alloc();
if (!audio_frame) {
printf("Could not allocate audio frame\n");
goto end;
}
audio_frame->format = audio_codec_ctx->sample_fmt;
audio_frame->channel_layout = av_get_default_channel_layout(AUDIO_CHANNELS);
audio_frame->nb_samples = audio_codec_ctx->frame_size;
audio_frame_size = av_samples_get_buffer_size(NULL, AUDIO_CHANNELS, audio_codec_ctx->frame_size, audio_codec_ctx->sample_fmt, 0);
audio_data = (uint8_t*)av_malloc(audio_frame_size);
// 3. 将JT1078协议的音频数据转换为PCM格式的音频数据。
// ...
// 4. 将PCM格式的音频数据送入音频编码器中,进行音频编码。
while (audio_data_size >= audio_frame_size) {
memcpy(audio_data, audio_data + audio_data_size - audio_frame_size, audio_frame_size);
audio_data_size -= audio_frame_size;
audio_frame->data[0] = audio_data;
audio_frame->pts = pts;
pts += audio_codec_ctx->frame_size;
ret = avcodec_send_frame(audio_codec_ctx, audio_frame);
if (ret < 0) {
printf("Error sending audio frame: %d\n", ret);
goto end;
}
while (ret >= 0) {
ret = avcodec_receive_packet(audio_codec_ctx, &audio_packet);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
}
else if (ret < 0) {
printf("Error encoding audio frame: %d\n", ret);
goto end;
}
audio_packet.stream_index = audio_stream->index;
audio_packet.pts = pts;
audio_packet.dts = pts;
audio_packet.duration = audio_codec_ctx->frame_size;
last_audio_pts = pts;
pts += audio_codec_ctx->frame_size;
ret = av_interleaved_write_frame(rtmp_fmt_ctx, &audio_packet);
av_packet_unref(&audio_packet);
if (ret < 0) {
printf("Error writing audio frame: %d\n", ret);
goto end;
}
audio_frame_count++;
}
}
// 5. 创建FFmpeg的RTMP推流上下文,设置RTMP推流地址。
avformat_alloc_output_context2(&rtmp_fmt_ctx, NULL, "flv", RTMP_URL);
if (!rtmp_fmt_ctx) {
printf("Could not create output context\n");
goto end;
}
rtmp_fmt = rtmp_fmt_ctx->oformat;
audio_stream = avformat_new_stream(rtmp_fmt_ctx, NULL);
if (!audio_stream) {
printf("Could not allocate audio stream\n");
goto end;
}
audio_stream->id = 0;
audio_codec_ctx->time_base = (AVRational){1, AUDIO_SAMPLE_RATE};
audio_stream->time_base = audio_codec_ctx->time_base;
ret = avcodec_parameters_from_context(audio_stream->codecpar, audio_codec_ctx);
if (ret < 0) {
printf("Could not copy audio codec parameters: %d\n", ret);
goto end;
}
ret = avio_open(&rtmp_fmt_ctx->pb, RTMP_URL, AVIO_FLAG_WRITE);
if (ret < 0) {
printf("Could not open output file: %d\n", ret);
goto end;
}
ret = avformat_write_header(rtmp_fmt_ctx, NULL);
if (ret < 0) {
printf("Error writing header to output file: %d\n", ret);
goto end;
}
// 6. 将音频编码后的数据通过RTMP推流上下文,推送到RTMP服务器上。
// ...
end:
if (audio_data) {
av_free(audio_data);
}
if (audio_frame) {
av_frame_free(&audio_frame);
}
if (audio_codec_ctx) {
avcodec_free_context(&audio_codec_ctx);
}
if (rtmp_fmt_ctx) {
av_write_trailer(rtmp_fmt_ctx);
if (!(rtmp_fmt->flags & AVFMT_NOFILE)) {
avio_closep(&rtmp_fmt_ctx->pb);
}
avformat_free_context(rtmp_fmt_ctx);
}
return 0;
}
```
注意:以上代码只是一个简单的示例,实际开发的时候需要根据具体需求进行修改和优化。
相关推荐
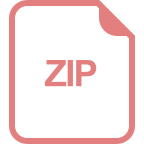














