将tensor格式转为字符串并保存在txt中
时间: 2023-06-01 21:06:53 浏览: 122
可以使用python中的numpy库将tensor格式转为字符串并保存在txt中,代码如下:
```python
import numpy as np
# 将tensor格式数据转为numpy数组
tensor_data = tensor_data.numpy()
# 将numpy数组转为字符串
str_data = np.array2string(tensor_data, separator=',')
# 写入txt文件
with open('data.txt', 'w') as f:
f.write(str_data)
```
相关问题
将tensor转为字符串并保存在txt中
可以使用numpy中的np.savetxt()函数将tensor转为字符串并保存在txt中。使用方法如下:
```
import numpy as np
# 定义一个tensor
tensor = np.array([[1, 2, 3], [4, 5, 6]])
# 将tensor转为字符串并保存在txt中
np.savetxt("tensor.txt", tensor, fmt="%d")
```
第二个参数"tensor"是要保存的tensor,第三个参数"fmt"指定了保存的数据格式,"%d"表示将数据保存为整型。保存后的txt文件内容如下:
```
1 2 3
4 5 6
```
将tensor转为字符串并保存
### 回答1:
您可以使用torch.save()函数将tensor保存为字符串:
```
import torch
tensor = torch.rand((3,3))
# 将tensor保存为字符串
str_tensor = torch.to_base64_string(tensor)
# 将字符串保存到文件中
with open('tensor.txt', 'w') as file:
file.write(str_tensor)
```
要将字符串转回为tensor,您可以使用torch.load()函数:
```
# 从文件中读取字符串
with open('tensor.txt', 'r') as file:
str_tensor = file.read()
# 将字符串转为tensor
tensor = torch.from_base64(str_tensor)
```
### 回答2:
要将 tensor 转为字符串并保存,可以使用以下步骤:
1. 首先,确保你已经安装了所需的 Python 模块。这些模块包括 TensorFlow 和 NumPy。可以使用以下命令安装它们:
```
pip install tensorflow numpy
```
2. 在代码中导入必要的库:
```python
import tensorflow as tf
import numpy as np
```
3. 创建一个 TensorFlow Session 并定义一个 tensor:
```python
# 创建 TensorFlow Session
sess = tf.Session()
# 定义一个 tensor
tensor = tf.constant([[1, 2, 3], [4, 5, 6]])
```
4. 使用 Session 的 `run` 方法计算 tensor 的值:
```python
# 计算 tensor 的值
tensor_value = sess.run(tensor)
```
5. 使用 NumPy 的 `array2string` 函数将 tensor 值转换为字符串。在此过程中,你还可以指定其他参数来定制字符串的格式,例如小数位数、分隔符等:
```python
# 将 tensor 值转为字符串
tensor_string = np.array2string(tensor_value, precision=2, separator=', ')
```
6. 最后,将 tensor 字符串保存到文件中:
```python
# 保存 tensor 字符串到文件
with open('tensor_string.txt', 'w') as file:
file.write(tensor_string)
```
通过以上步骤,你可以将 tensor 转为字符串并保存到文件中。请注意,在保存之前,确保已经使用 `session.run()` 方法计算出了 tensor 的值。
### 回答3:
将tensor转为字符串并保存需要按照以下步骤进行:
首先,引入相关的库,比如PyTorch或TensorFlow等库。
然后,创建一个tensor对象。
接下来,将tensor对象转化为numpy数组,可以使用tensor.numpy()方法。
然后可以使用numpy数组的tostring()方法将数组转为字符串。
最后,将字符串保存到文件中,可以使用Python的内置文件操作功能来实现。
下面是一个简单的代码示例:
```python
import torch
import numpy as np
# 创建一个tensor对象
tensor = torch.tensor([1, 2, 3])
# 将tensor转为numpy数组
array = tensor.numpy()
# 将数组转为字符串
string = array.tostring()
# 保存字符串到文件
with open("tensor_string.txt", "wb") as f:
f.write(string)
```
上面的代码中,首先创建一个简单的tensor对象,然后将其转为numpy数组,接着将数组转为字符串,最后使用文件操作功能将字符串保存到文件"tensor_string.txt"中。
希望这个回答对你有帮助。
阅读全文
相关推荐
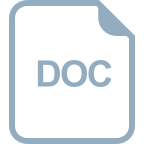
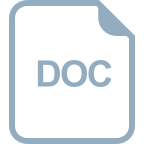
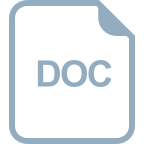
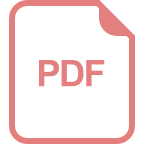
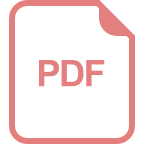
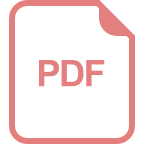
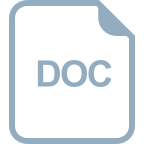
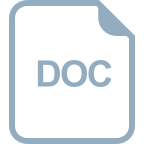
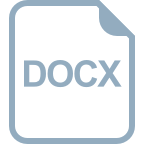
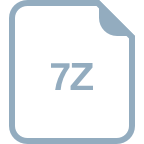
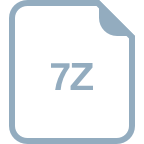
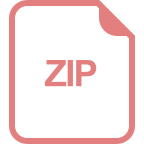
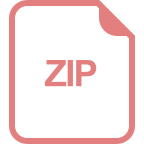