java链表的操作:1、构建空表H,插入 {a,b,,,f} 遍历输出单链表H 输出单链表H的长度
时间: 2023-12-17 07:05:33 浏览: 162
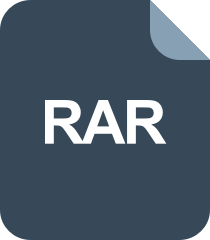
链表操作 java
Java中链表的操作可以通过LinkedList类实现。具体的操作如下:
1. 构建空表H
```java
LinkedList<String> H = new LinkedList<String>();
```
2. 插入 {a,b,,,f}
```java
H.add("a");
H.add("b");
H.add(null);
H.add(null);
H.add(null);
H.add("f");
```
注意:因为是单链表,所以需要使用add()方法进行尾部插入,而不能使用set()方法进行指定位置插入。
3. 遍历输出单链表H
```java
for(String s : H){
System.out.print(s + "->");
}
```
输出结果为:a->b->null->null->null->f->
4. 输出单链表H的长度
```java
System.out.println("链表H的长度为:" + H.size());
```
输出结果为:链表H的长度为:6
阅读全文
相关推荐
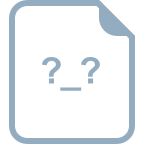



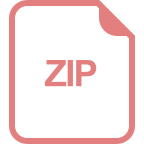
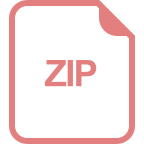
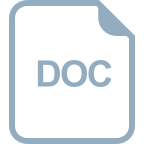
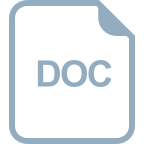
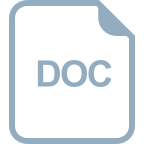
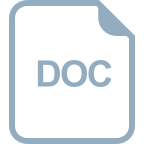
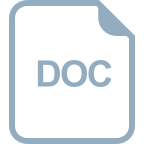
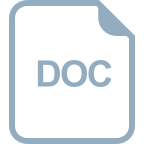
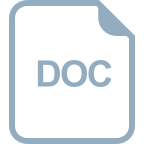
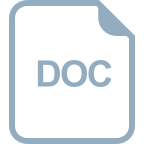
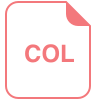
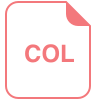
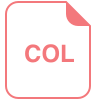