请用C语言编程:持续接收数据至缓存,每组数据长度为20,每10ms从缓存中提取最先存入的一组数据到buffer并将缓存中该组数据清除,数据缓存先进先出
时间: 2023-12-10 21:41:19 浏览: 70
以下是一个简单的示例代码,实现了持续接收数据至缓存,以及按照先进先出的顺序从缓存中提取数据到buffer:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <pthread.h>
#define BUFFER_SIZE 10 // 缓存大小
#define DATA_SIZE 20 // 数据长度
// 缓存结构体
struct buffer {
char data[BUFFER_SIZE][DATA_SIZE]; // 数据缓存
int head; // 缓存头指针
int tail; // 缓存尾指针
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t cond; // 条件变量
};
// 初始化缓存
void buffer_init(struct buffer *buf)
{
buf->head = 0;
buf->tail = 0;
pthread_mutex_init(&buf->mutex, NULL);
pthread_cond_init(&buf->cond, NULL);
}
// 往缓存中添加数据
void buffer_put(struct buffer *buf, const char *data)
{
pthread_mutex_lock(&buf->mutex);
// 如果缓存已满,等待空余位置
while ((buf->tail + 1) % BUFFER_SIZE == buf->head) {
pthread_cond_wait(&buf->cond, &buf->mutex);
}
// 添加数据
strcpy(buf->data[buf->tail], data);
buf->tail = (buf->tail + 1) % BUFFER_SIZE;
pthread_cond_signal(&buf->cond); // 通知等待读取的线程
pthread_mutex_unlock(&buf->mutex);
}
// 从缓存中取出数据
void buffer_get(struct buffer *buf, char *data)
{
pthread_mutex_lock(&buf->mutex);
// 如果缓存为空,等待有数据可读
while (buf->head == buf->tail) {
pthread_cond_wait(&buf->cond, &buf->mutex);
}
// 取出数据
strcpy(data, buf->data[buf->head]);
buf->head = (buf->head + 1) % BUFFER_SIZE;
pthread_cond_signal(&buf->cond); // 通知等待写入的线程
pthread_mutex_unlock(&buf->mutex);
}
// 线程函数,模拟数据接收
void *receive_thread(void *arg)
{
struct buffer *buf = (struct buffer *)arg;
char data[DATA_SIZE];
int count = 0;
while (1) {
sprintf(data, "data-%d", count++);
buffer_put(buf, data); // 添加数据到缓存
usleep(10000); // 模拟10ms延迟
}
return NULL;
}
int main()
{
struct buffer buf;
char data[DATA_SIZE];
buffer_init(&buf); // 初始化缓存
pthread_t tid;
pthread_create(&tid, NULL, receive_thread, &buf); // 创建数据接收线程
while (1) {
buffer_get(&buf, data); // 从缓存中取出数据
printf("%s\n", data);
}
return 0;
}
```
该程序使用了一个结构体来表示缓存,其中包含了一个字符数组用于存储数据,以及两个指针 head 和 tail 分别指向缓存的头和尾。当数据接收线程往缓存中添加数据时,tail 指针指向的位置就是新添加的数据的位置,添加完数据后 tail 指针向后移动一位。当主线程从缓存中取出数据时,head 指针指向的位置就是要取出的数据的位置,取出数据后 head 指针向后移动一位。如果 head 和 tail 指针相等,说明缓存为空。
为了实现先进先出的顺序,我们使用了互斥锁和条件变量来保证线程间的同步。当缓存已满时,数据接收线程会等待条件变量,直到有空余位置;当缓存为空时,主线程会等待条件变量,直到有数据可读。每次添加或取出数据后,都会通过条件变量通知等待的线程。
阅读全文
相关推荐
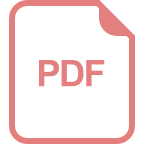
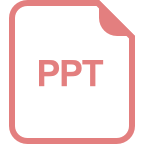
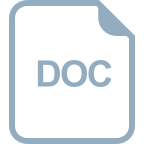
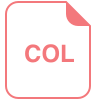













