用vue.js写一个发票表格
时间: 2023-07-18 13:10:35 浏览: 87
好的,以下是一个用 Vue.js 实现的发票表格示例代码:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Invoice Table</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</head>
<body>
<div id="app">
<table>
<thead>
<tr>
<th>发票号码</th>
<th>销售方名称</th>
<th>购买方名称</th>
<th>商品名称</th>
<th>数量</th>
<th>单价</th>
<th>金额</th>
<th>税率</th>
<th>税额</th>
<th>价税合计</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in invoiceItems" :key="index">
<td><input type="text" v-model="item.invoiceNo"></td>
<td><input type="text" v-model="item.sellerName"></td>
<td><input type="text" v-model="item.buyerName"></td>
<td><input type="text" v-model="item.productName"></td>
<td><input type="number" v-model="item.quantity"></td>
<td><input type="number" v-model="item.unitPrice"></td>
<td>{{ item.amount }}</td>
<td><input type="number" v-model="item.taxRate"></td>
<td>{{ item.taxAmount }}</td>
<td>{{ item.totalAmount }}</td>
</tr>
</tbody>
</table>
<button @click="addRow">添加行</button>
</div>
<script>
new Vue({
el: '#app',
data: {
invoiceItems: [
{ invoiceNo: '', sellerName: '', buyerName: '', productName: '', quantity: 0, unitPrice: 0, amount: 0, taxRate: 0, taxAmount: 0, totalAmount: 0 }
]
},
methods: {
addRow() {
this.invoiceItems.push({ invoiceNo: '', sellerName: '', buyerName: '', productName: '', quantity: 0, unitPrice: 0, amount: 0, taxRate: 0, taxAmount: 0, totalAmount: 0 });
}
},
computed: {
// 计算每条发票的金额、税额和价税合计
calculateAmount() {
this.invoiceItems.forEach(item => {
item.amount = item.quantity * item.unitPrice;
item.taxAmount = item.amount * item.taxRate;
item.totalAmount = item.amount + item.taxAmount;
});
}
}
});
</script>
</body>
</html>
```
这个示例代码中包括一个表格,表格中包含发票的各个字段,以及一个“添加行”按钮。通过 Vue.js 的 `v-for` 指令可以动态地生成多条发票记录。使用 `v-model` 指令可以将表单元素和 Vue 实例的数据进行双向绑定。在计算每条发票的金额、税额和价税合计时,使用了 Vue.js 中的计算属性(`computed`)来实现。
相关推荐
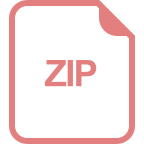
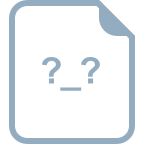














