如何结合switch和event code
时间: 2024-03-10 15:51:03 浏览: 51
可以将 `event.code` 的返回值作为 `switch` 语句的参数,然后在不同的 `case` 中进行相应的处理。以下是一个简单的示例:
```javascript
document.addEventListener('keydown', function(event) {
switch(event.code) {
case 'KeyA':
// 处理按下 A 键的情况
break;
case 'Enter':
// 处理按下 Enter 键的情况
break;
case 'ShiftLeft':
case 'ShiftRight':
// 处理按下左右 Shift 键的情况
break;
default:
// 处理其他按键的情况
break;
}
});
```
在这个示例中,我们注册了一个 `keydown` 事件监听器,当用户按下键盘上的任意一个键时,会触发这个事件。在事件处理函数中,我们根据按下的键的 `event.code` 值来判断用户按下了哪个键,然后在相应的 `case` 中进行处理。注意,我们可以在一个 `case` 中处理多个键,例如处理左右 Shift 键的情况。最后,我们可以在 `default` 分支中处理其他未被特别处理的按键。
相关推荐
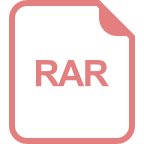
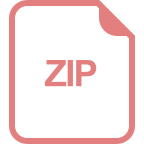
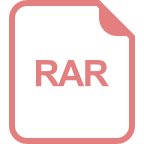
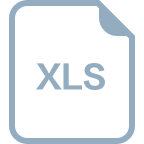
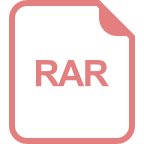
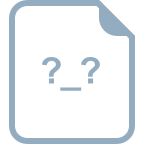
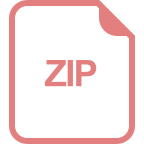
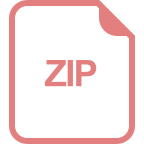
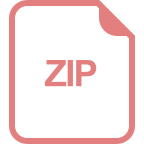
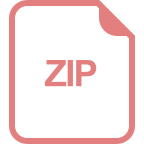
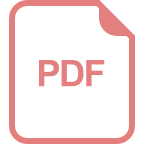
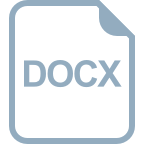
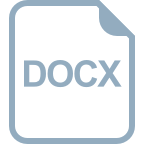
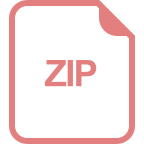