Write a piece of pseudo-code that override the onTouchEvent function in a View to perform “rotation” of the View content. You should use the appropriate event actions. No code needed for the object drawing.
时间: 2023-12-03 09:43:04 浏览: 31
Here's an example of pseudo-code that overrides the onTouchEvent function in a View to perform "rotation" of the View content:
```
@Override
public boolean onTouchEvent(MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
// Save the starting angle and coordinates of the touch event
mStartAngle = getAngle(event.getX(), event.getY());
mStartX = event.getX();
mStartY = event.getY();
return true;
case MotionEvent.ACTION_MOVE:
// Calculate the current angle of the touch event
float currentAngle = getAngle(event.getX(), event.getY());
// Calculate the difference between the starting and current angles
float angleDelta = currentAngle - mStartAngle;
// Rotate the View by the angle difference
rotate(angleDelta);
// Save the current angle as the starting angle for the next touch event
mStartAngle = currentAngle;
// Save the current coordinates as the starting coordinates for the next touch event
mStartX = event.getX();
mStartY = event.getY();
return true;
default:
return super.onTouchEvent(event);
}
}
private float getAngle(float x, float y) {
// Calculate the angle between the touch coordinates and the center of the View
float dx = x - getWidth() / 2f;
float dy = y - getHeight() / 2f;
return (float) Math.toDegrees(Math.atan2(dy, dx));
}
private void rotate(float angleDelta) {
// Rotate the View by the given angle difference
// No code needed for the object drawing
}
```
Note that this is just a rough example and may need to be adapted to fit your specific use case.
相关推荐
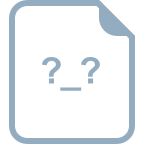
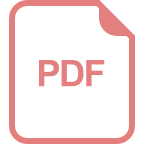
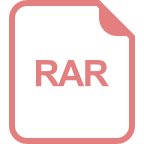














