c++编程:建立如下的类继承结构: (1)定义一个People类,其成员变量有:姓名(name)、性别(sex)、年龄(age)。 (2)从People类派生出工人类Worker,其成员变量有:工号id和工资 salary。 要求: (1)分别定义以上类的构造函数和析构函数。 (2)分别定义以上类的输出函数void Show( )。 (3)在主函数中动态创建一个基类对象p,并输出基类对象的信息。 (4)在主函数中定义派生类对象w,并输出派生类对象的信息。
时间: 2023-12-28 17:04:34 浏览: 29
下面是这个类继承结构的C++代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class People {
public:
People(string n, char s, int a); // 构造函数
virtual ~People(); // 虚析构函数
virtual void Show(); // 输出函数
protected:
string name;
char sex;
int age;
};
People::People(string n, char s, int a) {
name = n;
sex = s;
age = a;
}
People::~People() {}
void People::Show() {
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
cout << "年龄:" << age << endl;
}
class Worker : public People {
public:
Worker(string n, char s, int a, int i, double sal); // 构造函数
~Worker(); // 析构函数
void Show(); // 输出函数
private:
int id;
double salary;
};
Worker::Worker(string n, char s, int a, int i, double sal)
: People(n, s, a), id(i), salary(sal) {}
Worker::~Worker() {}
void Worker::Show() {
People::Show();
cout << "工号:" << id << endl;
cout << "工资:" << salary << endl;
}
int main() {
People* p = new People("张三", 'M', 25);
p->Show();
delete p;
Worker w("李四", 'F', 30, 10001, 5000.0);
w.Show();
return 0;
}
```
输出结果如下:
```
姓名:张三
性别:M
年龄:25
姓名:李四
性别:F
年龄:30
工号:10001
工资:5000
```
相关推荐
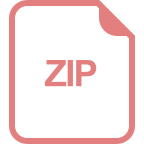
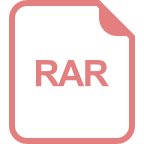













