棋盘覆盖Java代码
时间: 2024-10-30 16:07:05 浏览: 8
在计算机科学中,棋盘覆盖问题(如八皇后问题或汉诺塔问题)通常是关于在一个给定大小的棋盘上放置特定类型的元素,如皇后或圆盘,使得它们不会互相攻击或冲突。在Java中,你可以通过回溯算法、递归等方法来解决这类问题。
以下是一个简单的八皇后问题的Java代码示例,使用了递归方法:
```java
public class ChessBoardCover {
private static final int BOARD_SIZE = 8;
private boolean[] queens;
public void placeQueens() {
queens = new boolean[BOARD_SIZE];
solve(0);
}
private void solve(int row) {
if (row == BOARD_SIZE) {
printSolution();
return;
}
for (int col = 0; col < BOARD_SIZE; col++) {
if (!isSafe(row, col)) {
continue;
}
queens[row] = true;
solve(row + 1);
queens[row] = false; // 回溯:如果当前行无法放置,撤销并尝试下一个位置
}
}
private boolean isSafe(int row, int col) {
// 检查列、对角线是否安全
for (int i = 0; i < row; i++) {
if (queens[i] || row - i == col - i || row + i == col - i) {
return false;
}
}
return true;
}
private void printSolution() {
for (boolean queen : queens) {
System.out.print(queen ? "Q" : ".");
}
System.out.println();
}
public static void main(String[] args) {
ChessBoardCover boardCover = new ChessBoardCover();
boardCover.placeQueens();
}
}
```
在这个例子中,`placeQueens()` 方法开始放置皇后,`solve()` 函数递归地处理每一行,`isSafe()` 判断当前位置是否可以放置皇后,`printSolution()` 用于显示解决方案。
阅读全文
相关推荐
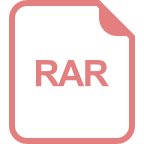
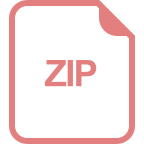
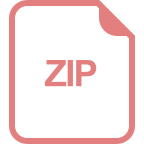
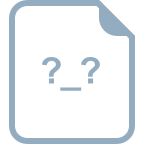
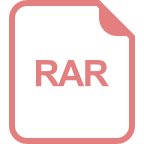
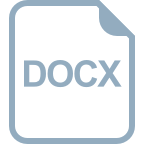
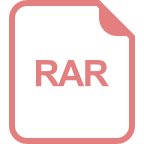
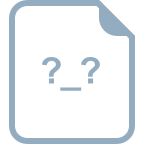
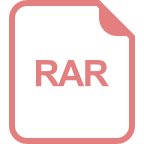
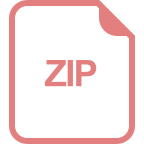
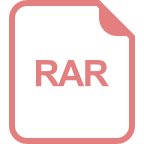
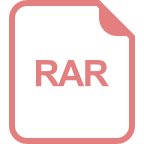
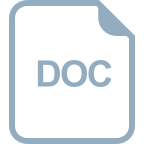
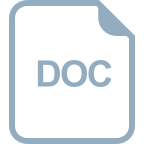

