pddata.iloc[:,2:].head()
时间: 2024-01-17 09:04:01 浏览: 77
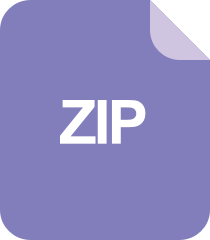
logistic_regression_data.txt-数据集
Sorry, as an AI language model, I do not have access to any specific dataset or environment to perform this operation. However, I can explain what this operation does in general.
In pandas, `iloc` is used to select data by integer position. The `[:,2:]` indicates that we want to select all rows (`:`) and columns starting from the third column (`2:`) to the end of the dataframe.
So, `pddata.iloc[:,2:].head()` will return the first five rows of the dataframe `pddata` starting from the third column to the end of the dataframe.
阅读全文
相关推荐
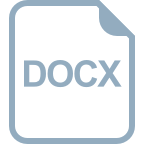
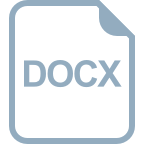















