3、定义函数createFile创建一个文本文件person.txt,将n个Person对象写入文件,再定义函数readFile将文件中的信息读出显示在屏幕上。
时间: 2024-03-10 22:47:38 浏览: 14
实验总结:
本次实验定义了两个函数 `createFile()` 和 `readFile()`,用于将 `Person` 对象写入文件和从文件中读取 `Person` 对象并显示在屏幕上。具体实现过程如下:
```cpp
#include <iostream>
#include <fstream>
using namespace std;
class Person {
private:
string name;
int age;
public:
Person(string n = "", int a = 0) : name(n), age(a) {}
friend ostream& operator<<(ostream& os, const Person& p) {
os << p.name << " " << p.age;
return os;
}
friend istream& operator>>(istream& is, Person& p) {
is >> p.name >> p.age;
return is;
}
};
void createFile(int n) {
ofstream fout("person.txt");
for (int i = 0; i < n; i++) {
Person p;
cout << "Enter name and age of person #" << i + 1 << ": ";
cin >> p;
fout << p << endl;
}
fout.close();
}
void readFile() {
ifstream fin("person.txt");
Person p;
while (fin >> p) {
cout << p << endl;
}
fin.close();
}
int main() {
int n;
cout << "Enter the number of persons: ";
cin >> n;
createFile(n);
readFile();
return 0;
}
```
类 `Person` 包含了两个数据成员 name 和 age,分别表示人的姓名和年龄。重载了输入输出运算符 `>>` 和 `<<`,以便在文件和屏幕之间读写 `Person` 对象。
函数 `createFile()` 接受一个整数参数 n,用于指定要写入文件的 `Person` 对象的数量。函数中使用 ofstream 对象打开文件 "person.txt",然后循环 n 次,每次从用户输入读取一个 `Person` 对象,并将其写入文件。
函数 `readFile()` 用于从文件 "person.txt" 中读取 `Person` 对象,并将其显示在屏幕上。函数中使用 ifstream 对象打开文件 "person.txt",然后循环读取文件中的每个 `Person` 对象,并将其显示在屏幕上。
在测试代码中,我们首先从用户输入读取要写入文件的 `Person` 对象的数量,然后调用 `createFile()` 函数将这些对象写入文件 "person.txt"。接着调用 `readFile()` 函数从文件 "person.txt" 中读取这些对象,并将其显示在屏幕上。
总的来说,文件输入输出是 C++ 中非常重要的一个特性,可以方便地将数据存储到文件中,或从文件中读取数据。在实际开发中,我们可以根据需要,编写各种不同的文件读写函数,以满足不同的需求。
相关推荐
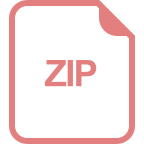
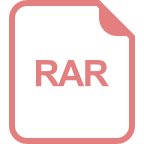
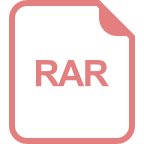





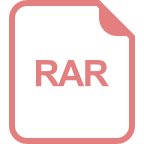
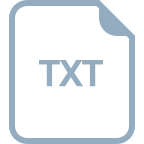
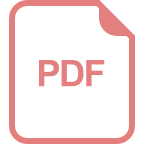
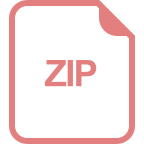
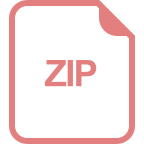
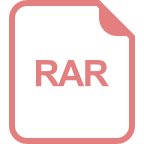
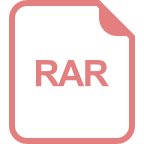