c++定义函数createFile创建一个文本文件person.txt,将n个Person对象写入文件,再定义函数readFile将文件中的信息读出显示在屏幕上。
时间: 2024-03-03 18:48:11 浏览: 156
好的,这个问题需要你提供Person对象的定义,以下是一个示例的Person类定义:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Person {
public:
Person(string name, int age, string address) {
this->name = name;
this->age = age;
this->address = address;
}
string getName() const { return name; }
int getAge() const { return age; }
string getAddress() const { return address; }
private:
string name;
int age;
string address;
};
```
现在我们可以定义createFile函数,将n个Person对象写入person.txt文件:
```c++
void createFile(int n) {
ofstream fout("person.txt");
if (!fout.is_open()) {
cout << "Failed to create file." << endl;
return;
}
for (int i = 0; i < n; i++) {
string name = "person" + to_string(i + 1);
int age = 20 + i;
string address = "address" + to_string(i + 1);
Person p(name, age, address);
fout << p.getName() << " " << p.getAge() << " " << p.getAddress() << endl;
}
fout.close();
}
```
接下来,我们可以定义readFile函数,从person.txt文件中读取信息并显示在屏幕上:
```c++
void readFile() {
ifstream fin("person.txt");
if (!fin.is_open()) {
cout << "Failed to open file." << endl;
return;
}
string name, address;
int age;
while (fin >> name >> age >> address) {
cout << "Name: " << name << ", Age: " << age << ", Address: " << address << endl;
}
fin.close();
}
```
现在我们可以在main函数中调用这两个函数,如下所示:
```c++
int main() {
int n = 5;
createFile(n);
readFile();
return 0;
}
```
这样就可以创建一个person.txt文件并将n个Person对象写入文件,再从文件中读取信息并显示在屏幕上了。
阅读全文
相关推荐





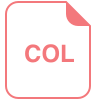
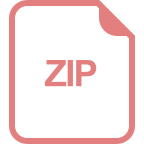
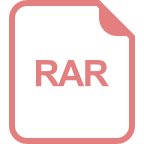