定义函数createFile创建一个文本文件person.txt,将n个Person对象写入文件,再定义函数readFile将文件中的信息读出显示在屏幕上c++
时间: 2024-03-10 11:47:26 浏览: 29
以下是一个示例代码,实现了创建一个文本文件并将Person对象写入,然后再从文件中读取数据并显示:
```c++
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
struct Person {
string name;
int age;
};
void createFile(string filename, int n) {
ofstream ofs(filename);
if (!ofs) {
cerr << "Failed to open file " << filename << endl;
return;
}
for (int i = 0; i < n; i++) {
Person p;
cout << "Enter name of person " << i+1 << ": ";
getline(cin, p.name);
cout << "Enter age of person " << i+1 << ": ";
cin >> p.age;
cin.ignore(); // ignore the newline character left in the input stream
ofs << p.name << " " << p.age << endl;
}
ofs.close();
}
void readFile(string filename) {
ifstream ifs(filename);
if (!ifs) {
cerr << "Failed to open file " << filename << endl;
return;
}
vector<Person> people;
string line;
while (getline(ifs, line)) {
Person p;
stringstream ss(line);
ss >> p.name >> p.age;
people.push_back(p);
}
ifs.close();
for (const auto& p : people) {
cout << "Name: " << p.name << ", Age: " << p.age << endl;
}
}
int main() {
createFile("person.txt", 3);
readFile("person.txt");
return 0;
}
```
在这个示例中,我们定义了一个结构体`Person`,其中包含了一个人的姓名和年龄。我们首先在`createFile`函数中创建一个文件并向其中写入`n`个`Person`对象,然后在`readFile`函数中从文件中读取数据并将其保存到一个`vector`中,最后在屏幕上显示出来。在`main`函数中,我们先调用`createFile`函数来创建文件并写入数据,然后再调用`readFile`函数来读取并显示数据。
相关推荐
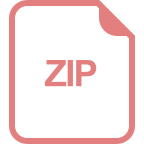
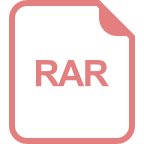
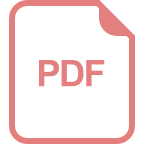






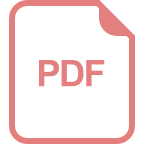
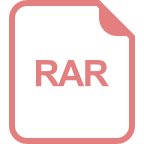
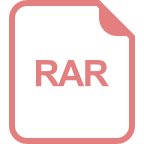
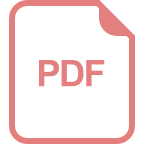
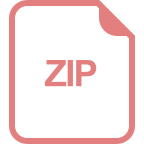
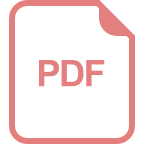