粒子群算法优化xgboost参数寻优代码
时间: 2023-06-30 21:26:37 浏览: 103
下面是使用 Python 实现粒子群算法优化 XGBoost 参数寻优的示例代码:
```python
import random
from xgboost import XGBClassifier
from sklearn.datasets import load_breast_cancer
from sklearn.model_selection import cross_val_score
# 定义超参数的搜索范围
learning_rate_range = (0.01, 0.1)
n_estimators_range = (50, 300)
max_depth_range = (3, 10)
subsample_range = (0.5, 0.8)
colsample_bytree_range = (0.5, 0.8)
lambda_range = (0.1, 10)
alpha_range = (0.1, 10)
# 定义 PSO 算法的参数
n_particles = 20 # 粒子数量
n_iterations = 50 # 迭代次数
w = 0.8 # 惯性因子
c1 = 2.0 # 个体学习因子
c2 = 2.0 # 全局学习因子
# 加载数据集
dataset = load_breast_cancer()
X, y = dataset.data, dataset.target
# 定义适应度函数
def fitness_function(position):
# 将超参数列表转换为字典形式
params = {'learning_rate': position[0],
'n_estimators': int(position[1]),
'max_depth': int(position[2]),
'subsample': position[3],
'colsample_bytree': position[4],
'lambda': position[5],
'alpha': position[6]}
# 构建 XGBoost 分类器
clf = XGBClassifier(**params)
# 使用交叉验证评估模型性能
scores = cross_val_score(clf, X, y, cv=5)
# 返回模型的平均准确率作为适应度函数值
return scores.mean()
# 初始化粒子群
particles = []
v = []
p_best = []
g_best = None
for i in range(n_particles):
# 随机生成一个超参数组合
position = [random.uniform(*r) for r in [learning_rate_range,
n_estimators_range,
max_depth_range,
subsample_range,
colsample_bytree_range,
lambda_range,
alpha_range]]
particles.append(position)
# 随机生成一个速度向量
v.append([random.uniform(-1, 1) for _ in range(len(position))])
# 记录当前最优位置
p_best.append(position)
if g_best is None or fitness_function(position) > fitness_function(g_best):
g_best = position
# 迭代优化
for t in range(n_iterations):
for i in range(n_particles):
# 更新速度
for j in range(len(particles[i])):
r1 = random.uniform(0, 1)
r2 = random.uniform(0, 1)
v[i][j] = w * v[i][j] + c1 * r1 * (p_best[i][j] - particles[i][j]) + c2 * r2 * (g_best[j] - particles[i][j])
# 更新位置
particles[i] = [particles[i][j] + v[i][j] for j in range(len(particles[i]))]
# 边界处理
particles[i] = [min(max(particles[i][j], r[0]), r[1]) for j, r in enumerate([learning_rate_range,
n_estimators_range,
max_depth_range,
subsample_range,
colsample_bytree_range,
lambda_range,
alpha_range])]
# 更新个体最优值
if fitness_function(particles[i]) > fitness_function(p_best[i]):
p_best[i] = particles[i]
# 更新全局最优值
if fitness_function(particles[i]) > fitness_function(g_best):
g_best = particles[i]
# 打印当前迭代的最优值
print('Iteration {}: Best Fitness = {:.4f}'.format(t, fitness_function(g_best)))
# 输出最优超参数组合
print('Best Parameters:', g_best)
# 训练一个新的模型并进行预测
clf = XGBClassifier(learning_rate=g_best[0], n_estimators=int(g_best[1]), max_depth=int(g_best[2]),
subsample=g_best[3], colsample_bytree=g_best[4], reg_lambda=g_best[5], reg_alpha=g_best[6])
clf.fit(X, y)
```
在这个示例中,我们使用 PSO 算法优化 XGBoost 模型的 7 个超参数,即学习率、树的数量、决策树的最大深度、样本采样方式、特征采样方式、L2 正则化参数和 L1 正则化参数。我们首先定义了每个超参数的搜索范围,然后使用交叉验证的方式评估每个超参数组合的性能,并将模型的平均准确率作为适应度函数的值。接着,我们使用 PSO 算法随机生成一定数量的粒子,并对每个粒子进行初始化。在迭代过程中,我们根据当前的适应度函数值和历史最优适应度函数值,更新每个粒子的位置和速度,以便于探索更优的超参数组合。最后,我们将历史最优的超参数组合作为 XGBoost 模型的最优超参数,并训练一个新的模型,用于最终的预测任务。
相关推荐
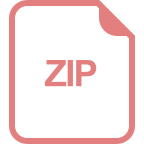
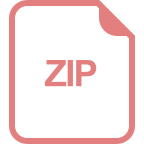
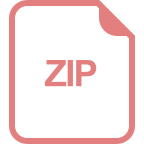














