c++tree结构使用简单例子
时间: 2024-01-07 16:06:04 浏览: 135
C++ STL 中的 `tree` 是一个非标准的容器,需要使用第三方库 `GCC-Tree` 才能使用。下面是一个简单的例子,演示如何使用 `tree` 存储一组整数,并对其进行遍历和查找操作:
```c++
#include <iostream>
#include <ext/pb_ds/assoc_container.hpp>
#include <ext/pb_ds/tree_policy.hpp>
using namespace std;
using namespace __gnu_pbds;
typedef tree<int, null_type, less<int>, rb_tree_tag, tree_order_statistics_node_update> ordered_set;
int main() {
ordered_set s;
s.insert(10);
s.insert(20);
s.insert(30);
s.insert(40);
s.insert(50);
cout << "size = " << s.size() << endl;
cout << "s[1] = " << *s.find_by_order(1) << endl;
cout << "s[2] = " << *s.find_by_order(2) << endl;
cout << "s.rank(30) = " << s.order_of_key(30) << endl;
cout << "s.rank(40) = " << s.order_of_key(40) << endl;
for (auto x : s) {
cout << x << " ";
}
return 0;
}
```
输出结果为:
```
size = 5
s[1] = 20
s[2] = 30
s.rank(30) = 2
s.rank(40) = 3
10 20 30 40 50
```
在这个例子中,我们首先定义了一个 `ordered_set` 类型的变量 `s`,它是一个存储整数类型元素的 `tree` 容器。然后,我们向 `s` 中插入了一些元素,使用 `size` 函数查看容器中元素的个数,使用 `find_by_order` 函数查找元素,使用 `order_of_key` 函数查找元素在容器中的排名,最后使用一个 for 循环遍历容器中的元素并输出。
需要注意的是,`tree` 是一个非标准的容器,使用时需要在编译选项中添加 `-std=c++11 -D_GLIBCXX_DEBUG`。此外,`tree` 容器的功能与 `set` 和 `map` 类似,但是由于 `tree` 使用的是红黑树,因此插入和删除操作的时间复杂度要比 `set` 和 `map` 高一些,但是查找操作的时间复杂度相对较低。
阅读全文
相关推荐
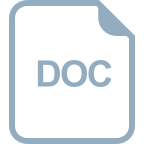
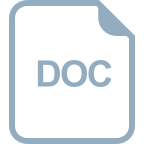
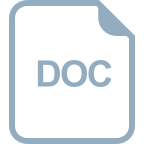
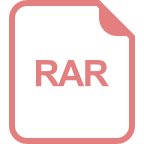
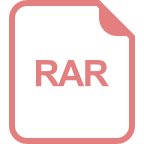
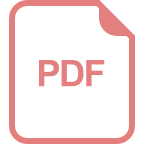
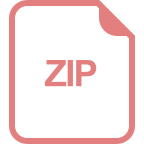
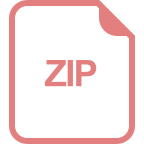
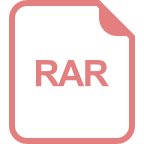
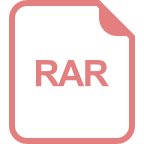
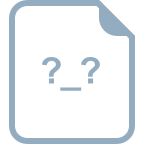
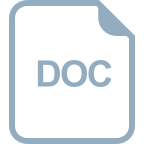
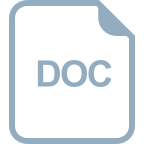
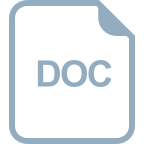
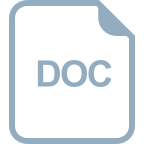
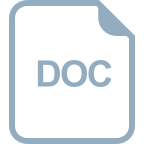
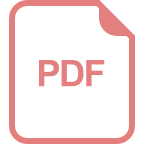
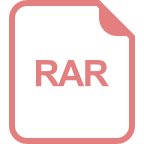
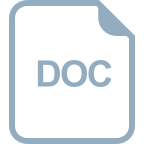