运行以下代码,页面中的输出结果是( )。 <div id="box"> <div v-if="score>=90">一等</div> <div v-else-if="score>=75">二等</div> <div v-else-if="score>=60">三等</div> <div v-else>四等</div> </div> <script type="text/javascript"> var demo = new Vue({ el : '#box', data : { score : 80 } }); </script>
时间: 2023-11-16 12:38:10 浏览: 93
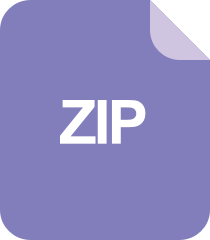
jQuery带评论的星星评分代码.zip
页面中的输出结果是 "二等"。
代码中使用了Vue的条件渲染指令v-if、v-else-if和v-else。根据数据中的score值,如果score>=90,则渲染div标签中的内容为"一等";如果score>=75,则渲染div标签中的内容为"二等";如果score>=60,则渲染div标签中的内容为"三等";否则,渲染div标签中的内容为"四等"。
在本例中,score的值为80,符合第二个判断条件,因此渲染的内容为"二等"。
阅读全文
相关推荐
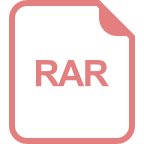
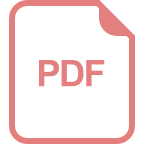
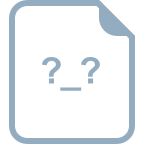
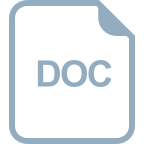
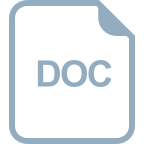












