hashmap获取第一个值
时间: 2023-08-06 07:04:19 浏览: 87
可以通过调用HashMap的values()方法获取所有的值,然后再调用Iterator的next()方法获取第一个值,如下所示:
```java
HashMap<String, String> map = new HashMap<>();
map.put("key1", "value1");
map.put("key2", "value2");
Iterator<String> iterator = map.values().iterator();
if (iterator.hasNext()) {
String firstValue = iterator.next();
System.out.println(firstValue);
}
```
运行结果为:value1
相关问题
java hashmap获取第一条数据
可以使用HashMap的keySet()方法获取所有的键集合,然后使用迭代器或者for-each循环遍历键集合,获取第一个键对应的值。示例代码如下:
```java
HashMap<String, String> hashMap = new HashMap<>();
hashMap.put("key1", "value1");
hashMap.put("key2", "value2");
hashMap.put("key3", "value3");
String firstValue = null;
for (String key : hashMap.keySet()) {
firstValue = hashMap.get(key);
break;
}
System.out.println("第一个值为:" + firstValue);
```
运行结果为:
```
第一个值为:value1
```
HASHmap 获取第一条数据和最后一条数据,用Java实现
可以使用Java中的HashMap类来实现。HashMap是一种基于哈希表实现的Map,它允许我们在O(1)的时间复杂度内进行数据的插入、查找和删除操作。
要获取第一条数据,可以使用HashMap的keySet()方法获取所有键的集合,然后再获取第一个键对应的值。代码如下:
```java
HashMap<String, String> map = new HashMap<String, String>();
// 往map中添加数据
map.put("key1", "value1");
map.put("key2", "value2");
map.put("key3", "value3");
// 获取第一条数据
String firstKey = map.keySet().iterator().next();
String firstValue = map.get(firstKey);
System.out.println("第一条数据为:" + firstKey + " -> " + firstValue);
```
要获取最后一条数据,可以使用HashMap的entrySet()方法获取所有键值对的集合,然后再获取最后一个键对应的值。代码如下:
```java
HashMap<String, String> map = new HashMap<String, String>();
// 往map中添加数据
map.put("key1", "value1");
map.put("key2", "value2");
map.put("key3", "value3");
// 获取最后一条数据
String lastKey = null;
String lastValue = null;
for (Map.Entry<String, String> entry : map.entrySet()) {
lastKey = entry.getKey();
lastValue = entry.getValue();
}
System.out.println("最后一条数据为:" + lastKey + " -> " + lastValue);
```
注意,HashMap并不保证元素的顺序,所以这种方法只适用于特定情况下的HashMap。如果需要按照插入顺序或其他顺序遍历HashMap,可以考虑使用LinkedHashMap。
阅读全文
相关推荐
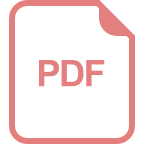
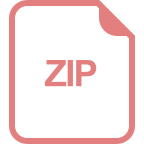
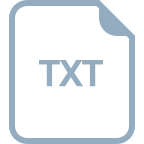
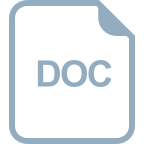










