timer =new QTimer(this); timer->start(500); connect(timer, &QTimer::timeout, this, &MainWindow::moveAgvs);},修改爲黨agv的current坐標都為整數型時,觸發moveagvs
时间: 2024-02-20 21:00:43 浏览: 158
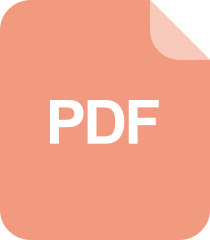
Qt基础开发之Qt多线程类QThread与Qt定时器类QTimer的详细方法与实例
如果将所有 AGV 的 current 坐标都改为整数型,可以修改成如下代码:
```
timer = new QTimer(this);
timer->start(500);
connect(timer, &QTimer::timeout, this, &MainWindow::moveAgvs);
```
这样,每隔 500ms 就会触发 `moveAgvs` 方法,进行 AGV 的移动。需要注意的是,由于坐标改为了整数型,可能会导致移动时出现卡顿或者抖动的情况,因此需要对代码进行一些优化,以确保 AGV 的移动效果流畅。例如可以尝试将 `setCurrentX` 和 `setCurrentY` 方法修改为整数型版本,或者在计算坐标时对其进行四舍五入操作,以尽可能减小误差。
阅读全文
相关推荐
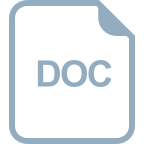
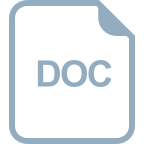











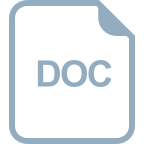
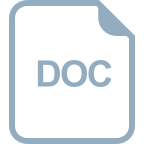
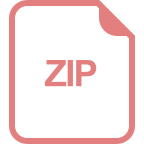
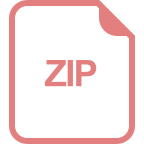